用python设计一个表示动物(Animal)的类,该类包括颜色(color)属性和叫(call)方法。再设计一个表示鱼(Fish)的类,包括尾巴(tail)和颜色(color)两个属性,及叫(call)方法。 提示:让Fish类继承自Animal类,重写__init__()和call方法。
时间: 2024-02-12 10:06:12 浏览: 118
好的,以下是用 Python 设计的动物类和鱼类:
```python
class Animal:
def __init__(self, color):
self.color = color
def call(self):
print("动物在叫")
class Fish(Animal):
def __init__(self, color, tail):
super().__init__(color)
self.tail = tail
def call(self):
print("鱼在叫")
```
在这个动物类中,我们定义了一个属性:颜色。然后,我们定义了一个方法:call(),用于表示动物的叫声。在鱼类中,我们使用继承来实现继承动物类的属性和方法。我们在鱼类中定义了两个属性:颜色和尾巴。我们重写了动物类的构造方法__init__(),并使用super()函数调用父类构造方法来初始化颜色属性。然后,我们还重写了动物类的call()方法,用于表示鱼的叫声。这样,我们就定义好了一个简单的动物类和鱼类。
相关问题
使用Python语言,设计一个表示动物的类,其内部有一个color属性和call方法
好的,以下是实现动物类的Python代码:
```python
class Animal:
def __init__(self, color):
self.color = color
def call(self):
raise NotImplementedError("Subclass must implement abstract method")
```
该类有一个构造函数 `__init__`,它接收一个颜色参数,并将其保存在成员变量 `color` 中。此外,还有一个抽象方法 `call`,需要在子类中实现。
你可以通过以下方式来创建一个子类 `Dog`:
```python
class Dog(Animal):
def call(self):
return "Woof!"
```
在这里,我们继承了 `Animal` 类,并实现了 `call` 方法。现在,我们可以创建一个 `Dog` 对象,并调用其 `call` 方法,如下所示:
```python
my_dog = Dog("brown")
print(my_dog.color) # 输出 "brown"
print(my_dog.call()) # 输出 "Woof!"
```
这样,我们就成功地创建了一个表示动物的类,并实现了其中的方法。
Python 设计一个表示动物(Animal)的类,该类包括颜色(color)属性和叫(call)方法。再设计一个表示鱼(Fish)的类,包括尾巴(tail)和颜色(color)两个属性,及叫(call)方法。
在Python中,我们可以先创建一个基本的`Animal`类,然后派生出更具体的子类`Fish`。下面是如何设计这两个类的例子:
```python
# 定义 Animal 类
class Animal:
def __init__(self, color):
self.color = color
# 定义叫声方法
def call(self):
raise NotImplementedError("Subclasses should implement this method.")
# 定义 Fish 类,继承自 Animal 类
class Fish(Animal):
def __init__(self, color, tail):
super().__init__(color)
self.tail = tail
# 实现叫声方法,这里是鱼类特有的叫声
def call(self):
return f"{self.color}的鱼说('swish')"
# 示例如何创建 Fish 对象
my_fish = Fish("蓝色", "长")
print(my_fish.call()) # 输出: 蓝色的鱼说('swish')
```
在这个例子中,`Animal`类有一个通用的颜色属性和抽象的`call()`方法,而`Fish`类继承了`Animal`,并且实现了`call()`方法,增加了特定于鱼的尾巴属性。当创建`Fish`实例并调用`call()`时,会输出鱼的叫声。
阅读全文
相关推荐
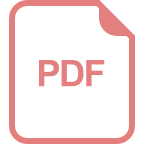
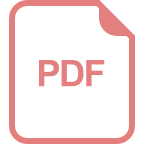
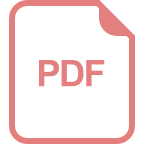








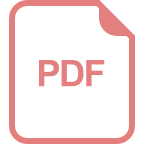
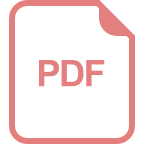
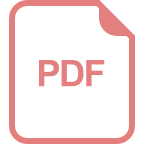
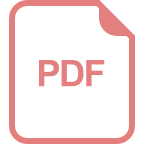
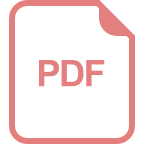
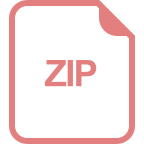