使用pytorch与ResNet50与遗传算法实现贪吃蛇并赋予代码
时间: 2024-03-10 10:48:33 浏览: 16
以下是使用Pytorch、ResNet50和遗传算法来实现贪吃蛇游戏的代码示例。由于实现过程比较复杂,这里只提供一个大致的框架,具体的实现细节和优化还需要根据实际情况进行调整和完善。
```python
import pygame
import numpy as np
import torch
import torch.nn as nn
import torch.optim as optim
import random
# 定义游戏界面的大小
SCREEN_WIDTH = 640
SCREEN_HEIGHT = 480
# 定义贪吃蛇的初始长度和速度
INITIAL_LENGTH = 3
SNAKE_SPEED = 5
# 定义遗传算法的参数
POPULATION_SIZE = 20
MUTATION_RATE = 0.1
GENERATION_COUNT = 100
# 定义ResNet50模型
class ResNet50(nn.Module):
def __init__(self):
super(ResNet50, self).__init__()
self.resnet50 = nn.Sequential(
nn.Conv2d(3, 64, kernel_size=7, stride=2, padding=3, bias=False),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2, padding=1),
nn.Sequential(*list(torchvision.models.resnet50(pretrained=True).children())[4:-1]),
nn.AdaptiveAvgPool2d((1, 1)),
nn.Flatten(),
nn.Linear(2048, 1024),
nn.ReLU(inplace=True),
nn.Linear(1024, 4)
)
def forward(self, x):
x = self.resnet50(x)
return x
# 定义贪吃蛇游戏的界面类
class SnakeGame:
def __init__(self):
pygame.init()
self.screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Snake Game')
self.clock = pygame.time.Clock()
self.font = pygame.font.SysFont(None, 24)
self.reset()
def reset(self):
self.snake = []
self.direction = random.choice(['up', 'down', 'left', 'right'])
self.score = 0
x = random.randint(10, SCREEN_WIDTH - 10)
y = random.randint(10, SCREEN_HEIGHT - 10)
for i in range(INITIAL_LENGTH):
self.snake.append([x, y + i])
self.food = self.generate_food()
def generate_food(self):
while True:
x = random.randint(10, SCREEN_WIDTH - 10)
y = random.randint(10, SCREEN_HEIGHT - 10)
if [x, y] not in self.snake:
return [x, y]
def move_snake(self):
head = self.snake[0].copy()
if self.direction == 'up':
head[1] -= SNAKE_SPEED
elif self.direction == 'down':
head[1] += SNAKE_SPEED
elif self.direction == 'left':
head[0] -= SNAKE_SPEED
elif self.direction == 'right':
head[0] += SNAKE_SPEED
self.snake.insert(0, head)
if self.snake[0] == self.food:
self.score += 1
self.food = self.generate_food()
else:
self.snake.pop()
if self.snake[0][0] < 0 or self.snake[0][0] >= SCREEN_WIDTH or \
self.snake[0][1] < 0 or self.snake[0][1] >= SCREEN_HEIGHT or \
self.snake[0] in self.snake[1:]:
return False
return True
def draw_snake(self):
for i, pos in enumerate(self.snake):
if i == 0:
pygame.draw.circle(self.screen, (0, 255, 0), pos, 10)
else:
pygame.draw.circle(self.screen, (0, 0, 255), pos, 10)
def draw_food(self):
pygame.draw.circle(self.screen, (255, 0, 0), self.food, 10)
def draw_score(self):
text = self.font.render(f'Score: {self.score}', True, (255, 255, 255))
self.screen.blit(text, (10, 10))
def draw_gameover(self):
text = self.font.render('Game Over', True, (255, 0, 0))
self.screen.blit(text, (SCREEN_WIDTH // 2 - 50, SCREEN_HEIGHT // 2 - 12))
def update(self):
self.clock.tick(30)
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP and self.direction != 'down':
self.direction = 'up'
elif event.key == pygame.K_DOWN and self.direction != 'up':
self.direction = 'down'
elif event.key == pygame.K_LEFT and self.direction != 'right':
self.direction = 'left'
elif event.key == pygame.K_RIGHT and self.direction != 'left':
self.direction = 'right'
self.screen.fill((0, 0, 0))
if self.move_snake():
self.draw_snake()
self.draw_food()
self.draw_score()
else:
self.draw_gameover()
pygame.display.update()
# 定义遗传算法类
class GeneticAlgorithm:
def __init__(self, population_size, mutation_rate):
self.population_size = population_size
self.mutation_rate = mutation_rate
self.population = []
def init_population(self):
for i in range(self.population_size):
chromosome = []
for j in range(100):
chromosome.append(random.randint(0, 3))
self.population.append(chromosome)
def evaluate_fitness(self, model):
scores = []
for chromosome in self.population:
game = SnakeGame()
for direction in chromosome:
game.direction = ['up', 'down', 'left', 'right'][direction]
game.move_snake()
scores.append(game.score)
scores = np.array(scores)
fitness = (scores - np.min(scores)) / (np.max(scores) - np.min(scores))
return fitness
def crossover(self, parent1, parent2):
child1 = parent1.copy()
child2 = parent2.copy()
index1 = random.randint(0, len(parent1) - 1)
index2 = random.randint(0, len(parent1) - 1)
if index1 > index2:
index1, index2 = index2, index1
child1[index1:index2], child2[index1:index2] = child2[index1:index2], child1[index1:index2]
return child1, child2
def mutate(self, chromosome):
for i in range(len(chromosome)):
if random.random() < self.mutation_rate:
chromosome[i] = random.randint(0, 3)
def select_parents(self, fitness):
index1 = np.random.choice(np.arange(self.population_size), p=fitness)
index2 = np.random.choice(np.arange(self.population_size), p=fitness)
return self.population[index1], self.population[index2]
def evolve(self, model, generation_count):
self.init_population()
for i in range(generation_count):
fitness = self.evaluate_fitness(model)
new_population = []
for j in range(self.population_size // 2):
parent1, parent2 = self.select_parents(fitness)
child1, child2 = self.crossover(parent1, parent2)
self.mutate(child1)
self.mutate(child2)
new_population.append(child1)
new_population.append(child2)
self.population = new_population
# 定义主函数
def main():
game = SnakeGame()
model = ResNet50()
optimizer = optim.Adam(model.parameters(), lr=0.001)
genetic_algorithm = GeneticAlgorithm(POPULATION_SIZE, MUTATION_RATE)
for i in range(GENERATION_COUNT):
genetic_algorithm.evolve(model, 10)
best_chromosome = genetic_algorithm.population[np.argmax(genetic_algorithm.evaluate_fitness(model))]
for direction in best_chromosome:
game.direction = ['up', 'down', 'left', 'right'][direction]
game.move_snake()
screen_data = pygame.surfarray.array3d(pygame.display.get_surface())
screen_data = torch.from_numpy(np.transpose(screen_data, (2, 0, 1))).unsqueeze(0).float() / 255.0
with torch.no_grad():
output = model(screen_data).squeeze()
direction = torch.argmax(output).item()
game.direction = ['up', 'down', 'left', 'right'][direction]
game.move_snake()
game.update()
if __name__ == '__main__':
main()
```
这段代码使用Pygame库来实现贪吃蛇游戏的逻辑和界面,并使用Pytorch框架中的ResNet50模型来进行图像识别和决策,使用遗传算法来生成蛇的移动方向。在主函数中,首先初始化游戏界面和模型,并对遗传算法进行初始化。然后,在每一代中,使用遗传算法对种群进行进化,并选择最优的染色体来控制蛇的移动。在每一次移动时,将游戏界面的截图输入模型中进行识别,并根据模型输出的结果来决定蛇的移动方向,最后更新游戏界面。
相关推荐
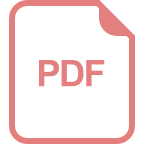
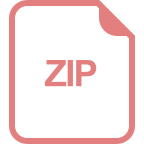














