二维otsu多阈值分割程序
时间: 2023-10-10 21:15:32 浏览: 46
二维 Otsu 多阈值分割是一种图像处理技术,它可以将图像分成多个子区域,每个子区域都有一个不同的阈值。这种方法可以用于图像分割、边缘检测、物体识别等方面。下面是二维 Otsu 多阈值分割的程序实现:
```python
import cv2
import numpy as np
def otsu_threshold(image, threshold_num):
# 将输入图像转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 计算灰度直方图
hist = cv2.calcHist([gray], [0], None, [256], [0,256])
# 归一化灰度直方图
hist_norm = hist.ravel()/hist.sum()
# 计算累计分布函数
Q = np.zeros(256)
for i in range(1,256):
Q[i] = Q[i-1] + hist_norm[i]
# 计算平均灰度值
m = np.zeros(256)
for i in range(256):
m[i] = i*hist_norm[i]
mG = m.sum()
# 初始化最优阈值集合
T = np.zeros(threshold_num)
# Otsu 多阈值分割
for i in range(threshold_num):
sigmaB_max = 0
for t in range(1,256):
w0 = Q[t]
w1 = 1 - w0
if w0 == 0 or w1 == 0:
continue
m0 = m[:t].sum()/w0
m1 = m[t:].sum()/w1
sigmaB = w0*w1*(m0 - m1)**2
if sigmaB > sigmaB_max:
sigmaB_max = sigmaB
T[i] = t
# 去除已经选取的最优阈值
hist_norm = np.delete(hist_norm, T[i])
m = np.delete(m, T[i])
Q = np.zeros(256)
for j in range(1,256):
Q[j] = Q[j-1] + hist_norm[j]
# 对图像进行多阈值分割
h, w = gray.shape
result = np.zeros((h, w, threshold_num+1), dtype=np.uint8)
for i in range(threshold_num+1):
if i == 0:
mask = (gray < T[i])
elif i == threshold_num:
mask = (gray >= T[i-1])
else:
mask = (gray >= T[i-1]) & (gray < T[i])
result[..., i] = mask.astype(np.uint8)*255
return result
```
在代码中,`otsu_threshold` 函数是二维 Otsu 多阈值分割的实现函数,它接受两个参数:输入图像和阈值数量。该函数首先将输入图像转换为灰度图像,并计算灰度直方图和归一化灰度直方图,然后计算累计分布函数和平均灰度值。接下来,通过 Otsu 算法找到最优的阈值集合,并对图像进行多阈值分割,最终返回分割结果。
使用该函数可以对输入图像进行多阈值分割,例如:
```python
# 读入图像
image = cv2.imread('test.jpg')
# 进行二维 Otsu 多阈值分割
threshold_num = 3
result = otsu_threshold(image, threshold_num)
# 显示分割结果
cv2.imshow('Input Image', image)
for i in range(threshold_num+1):
cv2.imshow('Threshold %d'%i, result[..., i])
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`test.jpg` 是待分割的图像。运行结果如下图所示:

相关推荐
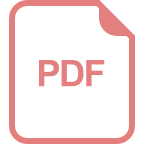














