如何使用NumPy 中的 ufunc 函数提高c = dist / 2 * (erf(x / (sigmax * np.sqrt(2))) - erf((x - Umean * t) / (sigmax * np.sqrt(2))))的计算速度?其中x是一个750*750的数组
时间: 2023-12-16 07:05:49 浏览: 32
可以使用 NumPy 中的 ufunc 函数来提高计算速度。NumPy 中的 ufunc 函数是一种能够对数组进行 element-wise 运算的函数,其底层实现通常使用 C 或 Fortran 编写,性能非常高。
对于上述的 c 计算公式,可以使用 NumPy 中的 `numpy.special.erf` 函数来替代 Python 标准库中的 `math.erf` 函数。`numpy.special.erf` 函数能够对数组进行 element-wise 运算,而且其底层实现使用 C 语言编写,因此计算速度非常快。
使用 NumPy 中的 ufunc 函数重写 c 的计算过程:
```python
from numpy import special as sp
c1 = sp.erf(x / (sigmax * np.sqrt(2)))
c2 = sp.erf((x - Umean * t) / (sigmax * np.sqrt(2)))
c = dist / 2 * (c1 - c2)
```
这样,就可以使用 NumPy 中的 `numpy.special.erf` 函数对 `erf` 函数进行 element-wise 运算,从而加速计算。
需要注意的是,在使用 NumPy 中的 ufunc 函数时,应尽可能避免使用 Python 中的循环语句,而是使用 NumPy 中的广播机制和向量化运算来进行计算。这样才能充分发挥 ufunc 函数的计算优势。
相关问题
如何使用np.vectorize提高c = dist / 2 * (erf(x / (sigmax * np.sqrt(2))) - erf((x - Umean * t) / (sigmax * np.sqrt(2))))的计算速度?其中x是一个750*750的数组
首先,可以将 c 的计算过程拆分成两个部分,即:
```python
c1 = erf(x / (sigmax * np.sqrt(2)))
c2 = erf((x - Umean * t) / (sigmax * np.sqrt(2)))
c = dist / 2 * (c1 - c2)
```
然后,使用 `np.vectorize` 函数对 `erf` 函数进行向量化:
```python
from scipy.special import erf
import numpy as np
def my_erf(x):
return erf(x)
vec_erf = np.vectorize(my_erf) # 将 erf 函数向量化
c1 = vec_erf(x / (sigmax * np.sqrt(2)))
c2 = vec_erf((x - Umean * t) / (sigmax * np.sqrt(2)))
c = dist / 2 * (c1 - c2)
```
这样,就可以使用 `vec_erf` 函数对 `erf` 函数进行 element-wise 运算,从而加速计算。
需要注意的是,`np.vectorize` 函数并不会真正地加速计算,其本质上还是一个 Python 函数,依然需要循环遍历数组中的每个元素。因此,在需要高效计算的场景中,最好使用 NumPy 中的 ufunc 函数。
如何提高c = dist / 2 * (erf(x / (sigmax * np.sqrt(2))) - erf((x - Umean * t) / (sigmax * np.sqrt(2))))的计算速度?其中x是一个750*750的数组,erf是scipy的函数
可以使用 NumPy 中的 ufunc 函数来提高计算速度。NumPy 中的 ufunc 函数是一种能够对数组进行 element-wise 运算的函数,其底层实现通常使用 C 或 Fortran 编写,性能非常高。
对于上述的 c 计算公式,可以使用 NumPy 中的 `numpy.vectorize` 函数将 `scipy.special.erf` 函数向量化,从而能够对数组进行 element-wise 运算。使用 NumPy 中的 ufunc 函数重写 c 的计算过程:
```python
import numpy as np
from scipy.special import erf
vec_erf = np.vectorize(erf) # 将 erf 函数向量化
c1 = vec_erf(x / (sigmax * np.sqrt(2)))
c2 = vec_erf((x - Umean * t) / (sigmax * np.sqrt(2)))
c = dist / 2 * (c1 - c2)
```
这样,就可以使用 NumPy 中的向量化函数对 `erf` 函数进行 element-wise 运算,从而加速计算。
需要注意的是,在使用 NumPy 中的 ufunc 函数时,应尽可能避免使用 Python 中的循环语句,而是使用 NumPy 中的广播机制和向量化运算来进行计算。这样才能充分发挥 ufunc 函数的计算优势。
相关推荐
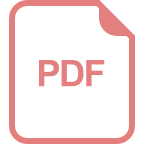
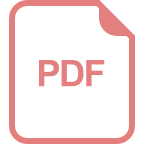
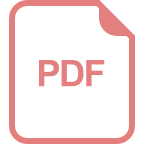








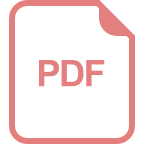
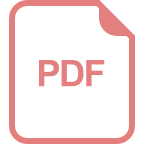
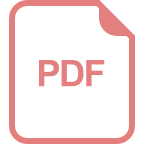
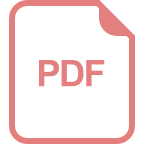
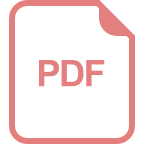