使用python将Excel中的指定sheet按照某一列分组,最上面插入一列红色底色白色字体加粗合并居中,第二列后按微软雅黑10号字体居中保存为不同的Excel文件
时间: 2023-06-20 17:06:21 浏览: 75
可以使用pandas和openpyxl库来实现,具体步骤如下:
1. 读取Excel文件并指定需要操作的sheet:
```python
import pandas as pd
df = pd.read_excel('example.xlsx', sheet_name='Sheet1')
```
2. 按照指定列分组:
```python
grouped = df.groupby('column_name')
```
3. 遍历分组结果并插入合并单元格的行:
```python
from openpyxl.styles import Font, PatternFill, Alignment
from openpyxl.utils import get_column_letter
from openpyxl import Workbook
for group_name, group_data in grouped:
wb = Workbook()
ws = wb.active
ws.title = group_name
# 插入合并单元格的行
ws.insert_rows(1)
ws.merge_cells(start_row=1, start_column=1, end_row=1, end_column=len(df.columns))
# 设置合并单元格的样式
font = Font(color='FFFFFFFF', bold=True)
fill = PatternFill(fill_type='solid', start_color='FF0000', end_color='FF0000')
alignment = Alignment(horizontal='center', vertical='center')
cell = ws.cell(row=1, column=1)
cell.font = font
cell.fill = fill
cell.alignment = alignment
# 设置第二列及以后列的样式
for col in range(2, len(df.columns)+1):
cell = ws.cell(row=1, column=col)
cell.font = Font(name='微软雅黑', size=10)
cell.alignment = Alignment(horizontal='center')
# 将分组结果写入Excel文件
for r in dataframe_to_rows(group_data, index=False, header=True):
ws.append(r)
wb.save(f'{group_name}.xlsx')
```
这样就可以按照指定列分组,并将每个分组的结果保存为不同的Excel文件。其中,需要替换掉代码中的`column_name`为指定的列名。
相关推荐
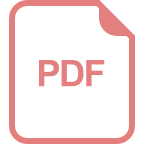
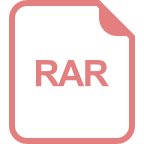














