设计并实现字符串的子字符串置换算法。给出c语言代码
时间: 2024-12-19 10:27:00 浏览: 31
设计一个C语言程序来实现字符串的子字符串置换算法,我们可以定义一个函数`replaceSubstrings()`,该函数接收两个字符串参数,一个是原始字符串`str1`,另一个是替换字符串`substitute`。函数的目标是查找`str1`中所有出现的`substitute`的子串,并将其替换成`substitute`本身。这里我们采用KMP算法(Knuth-Morris-Pratt算法)来查找匹配的子串。
以下是C语言代码实现:
```c
#include <stdio.h>
#include <string.h>
// KMP辅助函数,计算next数组
void computeNextArray(const char* pattern, int next[]) {
int len = strlen(pattern);
next[0] = -1;
int j = -1;
for (int i = 1; i < len; i++) {
while (j >= 0 && pattern[j + 1] != pattern[i]) {
j = next[j];
}
if (pattern[j + 1] == pattern[i]) {
j++;
}
next[i] = j;
}
}
// KMP搜索子串函数
bool kmpSearch(char* text, const char* pattern, int start) {
int text_len = strlen(text), pattern_len = strlen(pattern);
computeNextArray(pattern, next);
int j = 0;
for (int i = start; i < text_len; i++) {
while (j >= 0 && text[i] != pattern[j]) {
j = next[j];
}
if (text[i] == pattern[j]) {
j++;
}
if (j == pattern_len) {
printf("Substring found at position: %d\n", i - pattern_len + 1);
j = next[j]; // 移动指针到下一个位置
}
}
return j == -1; // 若遍历结束仍未找到匹配,则返回false
}
// 替换子字符串函数
void replaceSubstrings(char* str1, const char* substitute) {
int subLen = strlen(substitute);
for (int i = 0; ; i++) {
if (kmpSearch(str1 + i, substitute, i)) {
memmove(str1 + i + subLen, str1 + i, strlen(str1) - i); // 移动剩余部分
strcpy(str1 + i, substitute); // 将替换字符串插入
i += subLen - 1; // 更新起始位置,跳过已替换的部分
} else {
break; // 找不到更多子串了
}
}
}
int main() {
char str1[] = "Hello, how are you? This is a test.";
char substitute[] = "world";
printf("Original string: %s\n", str1);
replaceSubstrings(str1, substitute);
printf("After replacing substrings: %s\n", str1);
return 0;
}
```
在这个代码中,`computeNextArray()`计算了模式串的KMP表,`kmpSearch()`函数使用KMP表进行匹配查找,`replaceSubstrings()`则负责替换子字符串。当找到匹配子串时,会移动剩余部分并插入新的子串。
阅读全文
相关推荐
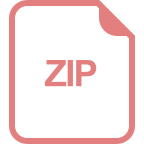
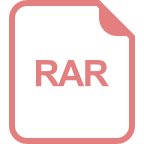
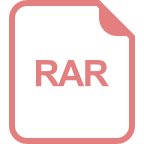
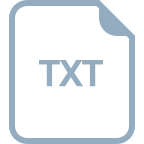
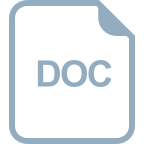
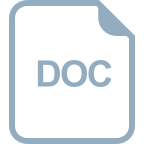
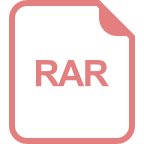
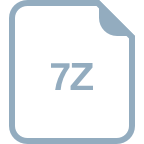
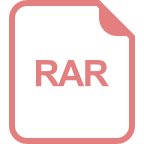
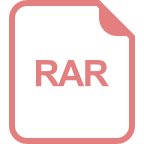
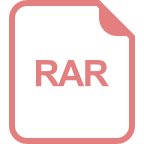
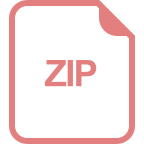
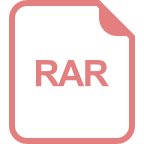
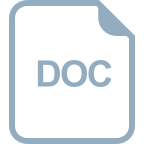
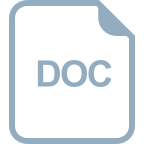
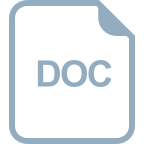
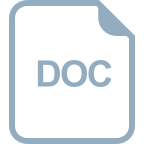
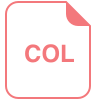