public void saveTheGameToFile(int index){ if(index<1||index>3){ System.out.println("Invalid save index:"+index); return; } String filename="save"+index+".dat";//存档的文件名 try(ObjectOutputStream output=new ObjectOutputStream(new FileOutputStream(filename))){ output.writeObject(board);//棋盘状态 output.writeInt();//玩家分数 output.writeInt(saveCount);//存档次数 output.writeChars();//玩家名字 output.writeInt();//玩家顺序 System.out.println("Successfully saved to file "+filename); }catch (IOException e){ System.out.println("Failed to save to file "+ filename); System.out.println("Sorry! Something went wrong: "+ e.getMessage()); } }解释以下这段代码的逻辑
时间: 2023-06-05 20:06:40 浏览: 55
这段代码定义了一个名为 "saveTheGameToFile" 的公共方法,该方法接收一个整数参数 index,用于指定要保存的游戏存档的索引。方法首先检查 index 是否有效,即在 1 到 3 之间,如果无效,则打印错误消息并返回。如果 index 有效,则使用该索引构造文件名,文件名的格式为 "save" + index + ".dat"。随后,使用 ObjectOutputStream 将棋盘状态、玩家分数、存档次数、玩家名称和玩家顺序写入到该文件中。如果保存成功,则输出成功消息,否则输出错误消息和异常信息。
相关问题
用双向链表作数据结构,用java语言编写一个通讯录管理系统,本系统应完成一下几方面的功能: 1)输入信息——enter(); 2)显示信息———display( ); 3)查找以姓名作为关键字 ———search( ); 4)删除信息———delete( ); 5)存盘———save ( ); 6)装入———load( ) ; 设计要求: 1)每条信息至包含 :姓名(NAME )街道(STREET)城市(CITY)邮编(EIP)国家(STATE)几项 2)作为一个完整的系统,应具有友好的界面和较强的容错能力,上机能正常运行 3)具有可视化界面
好的,我已经记录下来了你的问题。对于你的问题,我可以给出以下回答:
实现双向链表可以使用Java的LinkedList类,该类实现了List接口,同时也是一个双向链表。通讯录管理系统的实现可以借助该类来完成。
下面是一个简单的通讯录管理系统的代码示例:
```
import java.io.*;
import java.util.*;
class Contact {
String name;
String street;
String city;
int zip;
String state;
}
class ContactList {
LinkedList<Contact> contacts = new LinkedList<Contact>();
public void enter() {
Contact contact = new Contact();
Scanner scanner = new Scanner(System.in);
System.out.print("Enter name: ");
contact.name = scanner.nextLine();
System.out.print("Enter street: ");
contact.street = scanner.nextLine();
System.out.print("Enter city: ");
contact.city = scanner.nextLine();
System.out.print("Enter zip: ");
contact.zip = scanner.nextInt();
scanner.nextLine();
System.out.print("Enter state: ");
contact.state = scanner.nextLine();
contacts.add(contact);
}
public void display() {
for (Contact contact : contacts) {
System.out.println("Name: " + contact.name);
System.out.println("Street: " + contact.street);
System.out.println("City: " + contact.city);
System.out.println("Zip: " + contact.zip);
System.out.println("State: " + contact.state);
}
}
public void search(String name) {
boolean found = false;
for (Contact contact : contacts) {
if (contact.name.equals(name)) {
System.out.println("Name: " + contact.name);
System.out.println("Street: " + contact.street);
System.out.println("City: " + contact.city);
System.out.println("Zip: " + contact.zip);
System.out.println("State: " + contact.state);
found = true;
break;
}
}
if (!found) {
System.out.println("Contact not found.");
}
}
public void delete(String name) {
Iterator<Contact> iterator = contacts.iterator();
while (iterator.hasNext()) {
Contact contact = iterator.next();
if (contact.name.equals(name)) {
iterator.remove();
System.out.println("Contact deleted.");
return;
}
}
System.out.println("Contact not found.");
}
public void save() {
try {
ObjectOutputStream outputStream = new ObjectOutputStream(new FileOutputStream("contacts.dat"));
outputStream.writeObject(contacts);
outputStream.close();
System.out.println("Contacts saved.");
} catch (IOException e) {
System.out.println("Error saving contacts.");
}
}
public void load() {
try {
ObjectInputStream inputStream = new ObjectInputStream(new FileInputStream("contacts.dat"));
contacts = (LinkedList<Contact>)inputStream.readObject();
inputStream.close();
System.out.println("Contacts loaded.");
} catch (IOException e) {
System.out.println("Error loading contacts.");
} catch (ClassNotFoundException e) {
System.out.println("Error loading contacts.");
}
}
}
public class ContactManager {
public static void main(String[] args) {
ContactList contactList = new ContactList();
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("1. Enter contact");
System.out.println("2. Display contacts");
System.out.println("3. Search contacts");
System.out.println("4. Delete contact");
System.out.println("5. Save contacts");
System.out.println("6. Load contacts");
System.out.println("7. Exit");
System.out.print("Enter choice: ");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
contactList.enter();
break;
case 2:
contactList.display();
break;
case 3:
System.out.print("Enter name to search: ");
String name = scanner.nextLine();
contactList.search(name);
break;
case 4:
System.out.print("Enter name to delete: ");
name = scanner.nextLine();
contactList.delete(name);
break;
case 5:
contactList.save();
break;
case 6:
contactList.load();
break;
case 7:
System.exit(0);
break;
default:
System.out.println("Invalid choice.");
break;
}
}
}
}
```
该代码示例使用了一个Contact类来表示每个联系人,该类中包含了姓名、街道、城市、邮编和国家等信息。ContactList类则是一个管理联系人的类,它使用LinkedList来存储联系人列表,并提供了enter()、display()、search()、delete()、save()和load()等方法来进行联系人的添加、显示、查找、删除、存储和加载等操作。最后,ContactManager类是一个简单的控制台程序,它通过一个循环来接受用户的输入,并调用ContactList类的相应方法来完成操作。
写一个展示系统,要求以命令行的形式或图形的方式或界面的方式展示所有Java实验做的题目和运行结果。 改展示系统主要有下面的功能: 1、增加java实验的题目 2、修改题目 3、删除题目 4、为每个实验添加完成的程序 5、为每个实验添加完成的程序的运行结果 6、删除已提交的程序,删除已提交的结果 7、展示系统退出后提交的程序和结果保存在文件或数据库中。
这是一个相对复杂的系统,需要根据不同的需求和技术栈来实现。以下是一个简单的展示系统的示例代码,仅供参考。
```
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class JavaLabSystem {
private ArrayList<String> labTitles;
private Map<String, String> labPrograms;
private Map<String, String> labResults;
public JavaLabSystem() {
this.labTitles = new ArrayList<>();
this.labPrograms = new HashMap<>();
this.labResults = new HashMap<>();
}
public void addLab(String title, String program, String result) {
labTitles.add(title);
labPrograms.put(title, program);
labResults.put(title, result);
}
public void updateLab(String title, String program, String result) {
labPrograms.replace(title, program);
labResults.replace(title, result);
}
public void deleteLab(String title) {
labTitles.remove(title);
labPrograms.remove(title);
labResults.remove(title);
}
public void displayLabs() {
for (int i = 0; i < labTitles.size(); i++) {
System.out.println("[" + (i + 1) + "] " + labTitles.get(i));
}
}
public void displayLabDetails(String title) {
System.out.println("Title: " + title);
System.out.println("Program: " + labPrograms.get(title));
System.out.println("Result: " + labResults.get(title));
}
public void runLabProgram(String title) {
System.out.println("Running program for " + title + "...");
// TODO: 执行程序的代码
}
public void deleteLabProgram(String title) {
labPrograms.replace(title, "");
labResults.replace(title, "");
System.out.println("Program and result for " + title + " have been deleted.");
}
public void saveToFile() {
// TODO: 将数据保存到文件的代码
}
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
JavaLabSystem labSystem = new JavaLabSystem();
boolean isRunning = true;
while (isRunning) {
System.out.println("Java Lab System");
System.out.println("1. Add lab");
System.out.println("2. Update lab");
System.out.println("3. Delete lab");
System.out.println("4. Display labs");
System.out.println("5. Display lab details");
System.out.println("6. Run lab program");
System.out.println("7. Delete lab program");
System.out.println("8. Save to file");
System.out.println("9. Exit");
System.out.print("Enter your choice: ");
int choice = input.nextInt();
input.nextLine(); // 消耗掉回车符
switch (choice) {
case 1:
System.out.print("Enter lab title: ");
String title = input.nextLine();
System.out.print("Enter lab program: ");
String program = input.nextLine();
System.out.print("Enter lab result: ");
String result = input.nextLine();
labSystem.addLab(title, program, result);
System.out.println("Lab added successfully.");
break;
case 2:
System.out.print("Enter lab title to update: ");
String labTitle = input.nextLine();
if (labSystem.labTitles.contains(labTitle)) {
System.out.print("Enter new lab program: ");
String labProgram = input.nextLine();
System.out.print("Enter new lab result: ");
String labResult = input.nextLine();
labSystem.updateLab(labTitle, labProgram, labResult);
System.out.println("Lab updated successfully.");
} else {
System.out.println("Lab not found.");
}
break;
case 3:
System.out.print("Enter lab title to delete: ");
String labTitleToDelete = input.nextLine();
if (labSystem.labTitles.contains(labTitleToDelete)) {
labSystem.deleteLab(labTitleToDelete);
System.out.println("Lab deleted successfully.");
} else {
System.out.println("Lab not found.");
}
break;
case 4:
labSystem.displayLabs();
break;
case 5:
System.out.print("Enter lab title to display details: ");
String labTitleToDisplay = input.nextLine();
if (labSystem.labTitles.contains(labTitleToDisplay)) {
labSystem.displayLabDetails(labTitleToDisplay);
} else {
System.out.println("Lab not found.");
}
break;
case 6:
System.out.print("Enter lab title to run program: ");
String labTitleToRun = input.nextLine();
if (labSystem.labTitles.contains(labTitleToRun)) {
labSystem.runLabProgram(labTitleToRun);
} else {
System.out.println("Lab not found.");
}
break;
case 7:
System.out.print("Enter lab title to delete program: ");
String labTitleToDeleteProgram = input.nextLine();
if (labSystem.labTitles.contains(labTitleToDeleteProgram)) {
labSystem.deleteLabProgram(labTitleToDeleteProgram);
} else {
System.out.println("Lab not found.");
}
break;
case 8:
labSystem.saveToFile();
System.out.println("Data saved to file.");
break;
case 9:
isRunning = false;
System.out.println("Exiting Java Lab System...");
break;
default:
System.out.println("Invalid choice. Please try again.");
break;
}
System.out.println();
}
}
}
```
这个示例代码使用了面向对象的编程思想,将Java实验系统封装成了一个Java类,提供了增加、修改、删除、展示和运行实验等功能。这个示例代码仅供参考,实际开发中需要根据具体的需求进行修改和完善。
相关推荐
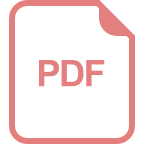
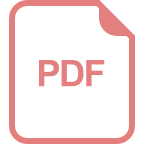
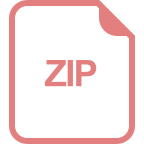












