创建一个多线程程序模拟两台抽奖机同时抽奖的情景。抽奖池用一个数组表示(int[] jiangjin = {0,0,0,0,0,0,0,0,0,0,10, 10, 10, 10, 10, 100, 100, 100,500, 500,1000};),创建两个抽奖箱(线程),设置线程名称分别为“1号抽奖箱”,“2号抽奖箱”分别进行抽奖,每0.1秒钟抽奖一次,每个抽奖箱抽奖5次,将抽奖结果在控制台输出。如下: 二号抽奖箱开出100元奖 一号抽奖箱:空奖,谢谢参与 二号抽奖箱:空奖,谢谢参与 一号抽奖箱:空奖,谢谢参与 一号抽奖箱开出10元奖 二号抽奖箱:空奖,谢谢参与 一号抽奖箱开出10元奖 二号抽奖箱开出800元奖 一号抽奖箱:空奖,谢谢参与 二号抽奖箱开出10元奖 一号抽奖箱抽奖结束 二号抽奖箱抽奖结束 提示:每张奖劵被开出后,就不能再次被开出了,可以考虑把抽出来这张从数组删除,或者设置一个标记。
时间: 2024-03-19 07:43:30 浏览: 154
可以按照以下代码实现:
```java
public class LotteryBox implements Runnable {
private static final int[] jiangjin = {0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 10, 10, 10, 10, 10, 100, 100, 100, 500, 500, 1000};
private static final int NUM_LOTTERIES = jiangjin.length;
private final String name;
private final int[] lotteryPool;
private final Random random;
private final int numLotteries;
private int numLotteriesLeft;
public LotteryBox(String name, int numLotteries) {
this.name = name;
this.numLotteries = numLotteries;
this.numLotteriesLeft = numLotteries;
this.lotteryPool = new int[NUM_LOTTERIES];
System.arraycopy(jiangjin, 0, this.lotteryPool, 0, NUM_LOTTERIES);
this.random = new Random();
}
private int drawLottery() {
int index = random.nextInt(NUM_LOTTERIES - numLotteriesLeft + 1);
int lottery = lotteryPool[index];
lotteryPool[index] = lotteryPool[NUM_LOTTERIES - numLotteriesLeft];
numLotteriesLeft--;
return lottery;
}
@Override
public void run() {
for (int i = 0; i < numLotteries; i++) {
int lottery = drawLottery();
String result = (lottery == 0) ? "空奖,谢谢参与" : "开出" + lottery + "元奖";
System.out.println(name + ":" + result);
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println(name + "抽奖结束");
}
}
public class Main {
public static void main(String[] args) {
LotteryBox box1 = new LotteryBox("1号抽奖箱", 5);
LotteryBox box2 = new LotteryBox("2号抽奖箱", 5);
Thread thread1 = new Thread(box1);
Thread thread2 = new Thread(box2);
thread1.start();
thread2.start();
}
}
```
在这段代码中,我们首先定义了一个 `LotteryBox` 类表示抽奖箱,它实现了 `Runnable` 接口,并重写了 `run()` 方法。在 `LotteryBox` 类中,我们维护了一个抽奖池 `lotteryPool`,它是一个长度为 `NUM_LOTTERIES` 的整数数组。其中 `NUM_LOTTERIES` 表示奖池中奖劵的数量。我们将每张奖劵的金额存储在数组中,其中前 10 张是空奖,后面的金额为 10 元、100 元、500 元和 1000 元。
在 `LotteryBox` 类中,我们实现了 `drawLottery()` 方法来模拟抽奖的过程。每次抽奖,我们使用 `Random` 类来生成一个随机数,然后从奖池中选取一张奖劵,将它的金额返回。同时,我们将这张奖劵从奖池中删除,以保证每张奖劵只能被开出一次。
在 `run()` 方法中,我们循环 5 次,每次调用 `drawLottery()` 方法来抽取一张奖劵,并将抽奖结果输出到控制台。同时,我们使用 `Thread.sleep()` 方法来模拟抽奖的时间间隔。抽奖结束后,我们输出一条消息表示抽奖箱已经结束抽奖。
在 `Main` 类中,我们创建了两个 `LotteryBox` 对象 `box1` 和 `box2`,并将它们分别传递给两个新线程 `thread1` 和 `thread2`。然后,我们调用 `thread1.start()` 和 `thread2.start()` 启动两个线程,让它们同时进行抽奖。
阅读全文
相关推荐
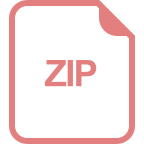

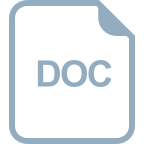
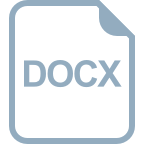
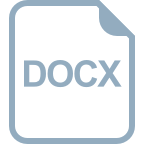
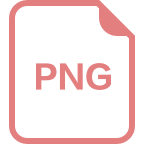

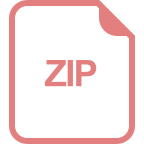
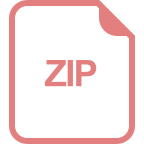
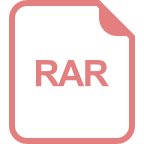
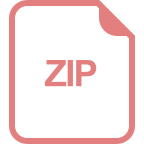
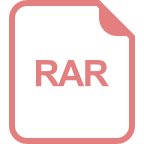
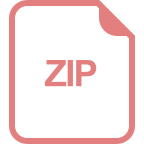