vue3实现tabs标签页
时间: 2023-09-13 18:05:52 浏览: 186
Vue3实现Tabs标签页可以使用组件化的思路,将每个标签页作为一个组件,再将所有标签页组合成一个Tabs组件。以下是一个简单的实现:
1. 创建Tabs组件
```
<template>
<div class="tabs">
<div class="tab-header">
<div v-for="(tab, index) in tabs" :key="index" :class="['tab-header-item', { 'active': currentIndex === index }]" @click="changeTab(index)">
{{ tab.title }}
</div>
</div>
<div class="tab-content">
<slot></slot>
</div>
</div>
</template>
<script>
export default {
name: 'Tabs',
data() {
return {
currentIndex: 0,
tabs: []
}
},
methods: {
changeTab(index) {
this.currentIndex = index
}
},
mounted() {
this.tabs = this.$children.map((component) => {
return {
title: component.title
}
})
}
}
</script>
<style>
.tabs .tab-header {
display: flex;
justify-content: space-between;
border-bottom: 1px solid #ccc;
}
.tabs .tab-header-item {
padding: 10px;
cursor: pointer;
}
.tabs .tab-header-item.active {
border-bottom: 2px solid #333;
}
.tabs .tab-content {
padding: 10px;
}
</style>
```
2. 创建Tab标签页组件
```
<template>
<div v-show="isActive">
<slot></slot>
</div>
</template>
<script>
export default {
name: 'Tab',
props: {
title: {
type: String,
required: true
}
},
data() {
return {
isActive: false
}
},
mounted() {
this.isActive = this.index === 0
},
computed: {
index() {
return this.$parent.tabs.length
}
}
}
</script>
```
3. 在Tabs组件中引用Tab标签页组件
```
<template>
<div>
<Tabs>
<Tab title="Tab 1">
<p>Tab 1 content</p>
</Tab>
<Tab title="Tab 2">
<p>Tab 2 content</p>
</Tab>
<Tab title="Tab 3">
<p>Tab 3 content</p>
</Tab>
</Tabs>
</div>
</template>
<script>
import Tabs from './Tabs.vue'
import Tab from './Tab.vue'
export default {
name: 'App',
components: {
Tabs,
Tab
}
}
</script>
```
以上代码实现了一个简单的Tabs标签页,可以通过修改样式和添加其他功能来满足实际需求。
阅读全文
相关推荐
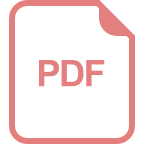















