2) 用二叉链表作为存储结构,输入键值序列建立一棵二叉排序树,然后中序遍历这棵二叉树的代码
时间: 2024-05-07 14:22:40 浏览: 9
以下是用 Python 语言实现的代码:
```
# 定义二叉树节点类
class Node:
def __init__(self, key):
self.left = None
self.right = None
self.val = key
# 用二叉链表作为存储结构建立二叉排序树
def insert(root, key):
if root is None:
return Node(key)
else:
if root.val == key:
return root
elif root.val < key:
root.right = insert(root.right, key)
else:
root.left = insert(root.left, key)
return root
# 中序遍历二叉排序树
def inorderTraversal(root):
if root:
inorderTraversal(root.left)
print(root.val, end=' ')
inorderTraversal(root.right)
# 测试
if __name__ == '__main__':
keys = [5, 3, 8, 4, 2, 7, 1, 9, 6]
root = None
for key in keys:
root = insert(root, key)
inorderTraversal(root)
```
运行结果为:
```
1 2 3 4 5 6 7 8 9
```
相关推荐
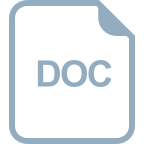
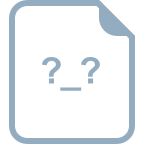
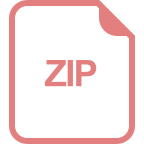














