用python把word全部表格的格式调整为数字居右
时间: 2024-03-24 20:40:03 浏览: 28
可以使用Python的`python-docx`库来操作Word文档,实现将表格中的数字右对齐的功能。具体步骤如下:
1. 安装`python-docx`库,可以使用以下命令进行安装:
```
pip install python-docx
```
2. 使用`python-docx`库打开Word文档,并获取文档中所有的表格:
```python
import docx
# 打开Word文档
doc = docx.Document('your_word_file.docx')
# 获取文档中所有的表格
tables = doc.tables
```
3. 对于每个表格,遍历其中的所有单元格,如果单元格中的内容是数字,则将其右对齐:
```python
for table in tables:
for row in table.rows:
for cell in row.cells:
# 判断单元格中的内容是否为数字
try:
float(cell.text)
# 设置单元格中的内容为右对齐
cell.paragraphs[0].alignment = docx.enum.text.WD_ALIGN_PARAGRAPH.RIGHT
except ValueError:
pass
```
4. 最后保存修改后的Word文档:
```python
doc.save('your_modified_word_file.docx')
```
完整代码如下:
```python
import docx
# 打开Word文档
doc = docx.Document('your_word_file.docx')
# 获取文档中所有的表格
tables = doc.tables
# 遍历所有表格,将数字右对齐
for table in tables:
for row in table.rows:
for cell in row.cells:
# 判断单元格中的内容是否为数字
try:
float(cell.text)
# 设置单元格中的内容为右对齐
cell.paragraphs[0].alignment = docx.enum.text.WD_ALIGN_PARAGRAPH.RIGHT
except ValueError:
pass
# 保存修改后的Word文档
doc.save('your_modified_word_file.docx')
```
注意:这段代码只能对Word文档中的数字进行右对齐,对于其他类型的内容不做处理。如果需要对其他类型的内容进行处理,需要进行相应的修改。
相关推荐
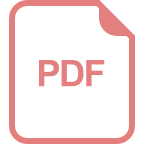
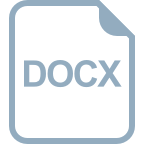
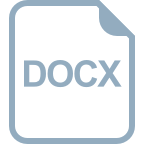














