2.js 创建一个单链表,把 1-100 中的质数存放到一个单链表中去。
时间: 2024-04-29 15:22:31 浏览: 86
好的,这个问题是关于编程实现的,我可以为你提供代码示例。以下是使用 JavaScript 实现一个单链表,将 1-100 中的质数存储到该单链表中的示例代码:
```javascript
// 定义一个单链表节点
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
// 定义一个单链表类
class LinkedList {
constructor() {
this.head = null;
this.tail = null;
this.length = 0;
}
// 添加节点到链表末尾
append(value) {
const node = new Node(value);
if (!this.head) {
this.head = node;
this.tail = node;
} else {
this.tail.next = node;
this.tail = node;
}
this.length++;
}
}
// 判断是否为质数的函数
function isPrime(n) {
if (n <= 1) return false;
for (let i = 2; i <= Math.sqrt(n); i++) {
if (n % i === 0) return false;
}
return true;
}
// 创建单链表,在其中存储 1-100 中的质数
const linkedList = new LinkedList();
for (let i = 1; i <= 100; i++) {
if (isPrime(i)) {
linkedList.append(i);
}
}
```
运行上述代码后,我们就可以在 `linkedList` 中存储 1-100 中的质数了。
阅读全文
相关推荐
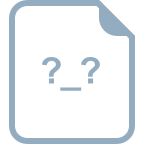

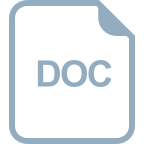
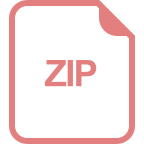



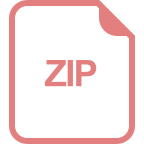
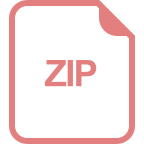
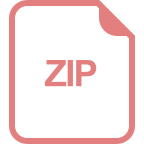
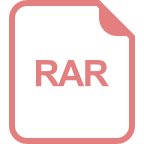
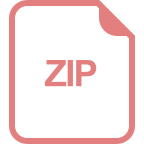
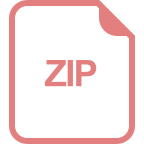