保存数据到vsc文件
时间: 2023-10-13 14:06:11 浏览: 107
如果你想将数据保存到VSCode项目中的文件中,可以使用以下步骤:
1. 创建一个文件夹来存储数据文件。
2. 在VSCode中右键点击该文件夹并选择“New File”选项,创建一个新的文件。
3. 在新文件中写入数据,并保存该文件。
4. 如果你需要在代码中读取该文件,可以使用以下代码:
```python
with open('path/to/file', 'r') as f:
data = f.read()
```
其中,'path/to/file'是文件的路径,'r'表示以只读方式打开文件。读取文件后,可以使用变量'data'来访问文件中的内容。
如果你需要在代码中写入数据到该文件中,可以使用以下代码:
```python
with open('path/to/file', 'w') as f:
f.write(data)
```
其中,'path/to/file'是文件的路径,'w'表示以写入方式打开文件。你可以使用变量'data'来写入数据到文件中。注意,这将覆盖文件中的所有内容,因此请谨慎使用。
相关问题
Python讲频谱仪的VSC数据保存到本地?
Python是一种非常流行的编程语言,可以用于许多领域,包括信号处理和频谱分析。要将VSC数据保存到本地,可以使用Python中的numpy和matplotlib库来进行处理和可视化。
首先,您需要使用VSC设备来获取数据并将其存储为CSV文件。然后,您可以使用Python的numpy库读取CSV文件中的数据,并使用matplotlib库将其绘制成图形。
以下是一个简单的Python代码示例,演示如何将VSC数据保存到本地:
```python
import numpy as np
import matplotlib.pyplot as plt
# 读取CSV文件中的数据
data = np.loadtxt('vsc_data.csv', delimiter=',')
# 绘制频谱图
plt.plot(data[:, 0], data[:, 1])
plt.xlabel('Frequency (Hz)')
plt.ylabel('Amplitude (dB)')
plt.title('VSC Spectrum')
plt.savefig('vsc_spectrum.png')
# 保存数据到Numpy格式文件
np.save('vsc_data.npy', data)
```
上述代码将读取名为“vsc_data.csv”的CSV文件,并将其绘制为频谱图。然后,它将图形保存为名为“vsc_spectrum.png”的PNG文件,并使用numpy库将数据保存为名为“vsc_data.npy”的Numpy格式文件。
FER2013.vsc数据集转化为YOLO格式
要将FER2013.vsc数据集转化为YOLO格式,可以按照以下步骤进行:
1. **了解数据集结构**:
- FER2013.vsc数据集通常包含图像文件和对应的标签。标签可能是情绪类别(如快乐、悲伤、愤怒等)。
2. **准备YOLO格式**:
- YOLO格式的标签文件是纯文本文件,每行表示一个对象,格式如下:
```
<object-class> <x_center> <y_center> <width> <height>
```
其中,`object-class`是类别的索引,`x_center`和`y_center`是对象边界框的中心坐标,`width`和`height`是边界框的宽度和高度。这些坐标是相对于图像宽度和高度的归一化值(范围在0到1之间)。
3. **转换步骤**:
- 遍历FER2013.vsc数据集中的每个图像文件。
- 读取图像和对应的标签。
- 将标签转换为YOLO格式的边界框坐标。
- 将转换后的标签保存为与图像文件同名的`.txt`文件。
4. **示例代码**:
```python
import os
import numpy as np
# 定义类别
classes = ['Angry', 'Disgust', 'Fear', 'Happy', 'Sad', 'Surprise', 'Neutral']
class_to_idx = {cls: idx for idx, cls in enumerate(classes)}
# 图像和标签的路径
image_dir = 'path_to_fer2013_images'
label_dir = 'path_to_fer2013_labels'
yolo_label_dir = 'path_to_save_yolo_labels'
# 创建YOLO标签目录
os.makedirs(yolo_label_dir, exist_ok=True)
# 遍历每个图像文件
for filename in os.listdir(image_dir):
if filename.endswith('.jpg') or filename.endswith('.png'):
image_path = os.path.join(image_dir, filename)
label_filename = os.path.splitext(filename)[0] + '.txt'
label_path = os.path.join(label_dir, label_filename)
yolo_label_path = os.path.join(yolo_label_dir, label_filename)
# 读取标签
with open(label_path, 'r') as f:
lines = f.readlines()
yolo_labels = []
for line in lines:
parts = line.strip().split()
class_name = parts[0]
x, y, w, h = map(float, parts[1:5])
# 转换为YOLO格式
x_center = x + w / 2
y_center = y + h / 2
width = w
height = h
yolo_labels.append(f"{class_to_idx[class_name]} {x_center} {y_center} {width} {height}")
# 保存YOLO标签
with open(yolo_label_path, 'w') as f:
f.write('\n'.join(yolo_labels))
```
5. **注意事项**:
- 确保类别名称和索引一致。
- 调整路径以匹配你的文件结构。
- 验证转换后的标签文件是否正确。
阅读全文
相关推荐



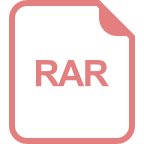












