编写一个程序,它首先打开一个文件,然后利用fork()创建一个子进程;随后,当父进程运行时先执行wait();父子进程都打印自己和其父进程的ID号;并且,二者都向该文件写入(利用write)一条信息,表明是在哪个进程中。用C语言
时间: 2024-02-18 11:59:52 浏览: 30
好的,以下是你需要的 C 语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
#include <sys/types.h>
#include <fcntl.h>
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s filename\n", argv[0]);
exit(1);
}
int fd = open(argv[1], O_CREAT | O_WRONLY | O_TRUNC, 0666);
if (fd == -1) {
printf("Failed to open file\n");
exit(1);
}
pid_t pid = fork();
if (pid == -1) {
printf("Failed to fork\n");
exit(1);
}
if (pid == 0) {
// 子进程
printf("Child process: pid=%d, ppid=%d\n", getpid(), getppid());
char *message = "This message is from child process\n";
write(fd, message, strlen(message));
} else {
// 父进程
wait(NULL);
printf("Parent process: pid=%d, ppid=%d\n", getpid(), getppid());
char *message = "This message is from parent process\n";
write(fd, message, strlen(message));
}
close(fd);
return 0;
}
```
在命令行中编译该程序时,可以使用以下命令:
```
gcc myfile.c -o myfile
```
在命令行中执行该程序时,可以指定要写入的文件名作为参数。例如:
```
./myfile output.txt
```
该程序将会创建一个名为 `output.txt` 的文件,并在其中写入子进程和父进程的信息。其中,子进程写入的信息是 "This message is from child process",父进程写入的信息是 "This message is from parent process"。
相关推荐
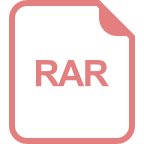
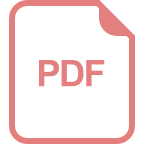
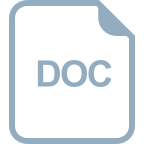














