springboot中使用mybatis实现动态sql同时使用mybatisPlus实现分页的详细流程
时间: 2024-03-27 14:39:33 浏览: 28
使用SpringBoot、MyBatis和MyBatis-Plus实现动态SQL和分页的详细流程如下:
1. 添加依赖
在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.3.1</version>
</dependency>
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper</artifactId>
<version>5.2.0</version>
</dependency>
```
2. 配置数据源
在application.properties文件中配置数据源信息,例如:
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT%2B8
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
```
3. Mapper文件
在Mapper文件中编写动态SQL的语句,例如:
```xml
<select id="queryByCondition" parameterType="map" resultMap="BaseResultMap">
select * from user
<where>
<if test="name != null and name != ''">
and name like concat('%', #{name}, '%')
</if>
<if test="age != null">
and age = #{age}
</if>
</where>
</select>
```
4. 继承BaseMapper
Mapper接口继承BaseMapper,例如:
```java
public interface UserMapper extends BaseMapper<User> {
List<User> queryByCondition(Map<String, Object> paramMap);
}
```
5. Service层
在Service层中调用Mapper层的方法,例如:
```java
public IPage<User> queryByCondition(String name, Integer age, Integer pageNum, Integer pageSize) {
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
if (StringUtils.isNotBlank(name)) {
queryWrapper.like("name", name);
}
if (age != null) {
queryWrapper.eq("age", age);
}
Page<User> page = new Page<>(pageNum, pageSize);
return userMapper.selectPage(page, queryWrapper);
}
```
6. Controller层
在Controller层中调用Service层的方法,例如:
```java
@GetMapping("/queryByCondition")
public Result queryByCondition(String name, Integer age, Integer pageNum, Integer pageSize) {
IPage<User> page = userService.queryByCondition(name, age, pageNum, pageSize);
return Result.success(page);
}
```
至此,SpringBoot、MyBatis和MyBatis-Plus实现动态SQL和分页的流程已经介绍完毕。
相关推荐
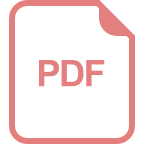
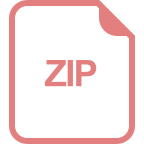














