请实现一个函数,把字符串 s 中的每个空格替换成"%20"。用c语言完成
时间: 2023-11-28 14:48:52 浏览: 33
可以使用类似于双指针的方法来实现:
```c
void replaceSpace(char* s, int length) {
// 计算替换后的长度
int count = 0;
for(int i = 0; i < length; i++) {
if(s[i] == ' ') {
count++;
}
}
int newLength = length + count * 2;
// 从后往前替换
int i = length - 1, j = newLength - 1;
while(i >= 0 && j > i) {
if(s[i] == ' ') {
s[j--] = '0';
s[j--] = '2';
s[j--] = '%';
} else {
s[j--] = s[i];
}
i--;
}
}
```
该函数会修改原字符串,将空格替换为"%20"。需要注意的是,如果替换后的字符串长度大于原字符串长度,需要确保原字符串有足够的空间。
相关问题
用C语言编写程序,将一个字符串拷贝到另一个字符串中,拷贝过程将空格替换成“%20”。
好的,可以使用如下的代码来实现:
```c
#include <stdio.h>
#include <string.h>
void replaceSpace(char *str, int length) {
int spaceCount = 0;
int i;
for (i = 0; i < length; i++) {
if (str[i] == ' ') {
spaceCount++;
}
}
int newLength = length + spaceCount * 2;
int indexOld = length - 1;
int indexNew = newLength - 1;
while (indexOld >= 0 && indexOld < indexNew) {
if (str[indexOld] == ' ') {
str[indexNew--] = '0';
str[indexNew--] = '2';
str[indexNew--] = '%';
} else {
str[indexNew--] = str[indexOld];
}
indexOld--;
}
}
int main() {
char str[100] = "hello world";
replaceSpace(str, strlen(str));
printf("%s\n", str);
return 0;
}
```
这个程序中,我们定义了一个 `replaceSpace` 函数,用来将字符串中的空格替换成 `%20`。具体思路是,首先遍历一遍字符串,统计出空格的数量,然后计算出替换后的字符串长度。之后,从字符串的末尾开始遍历,遇到空格就替换为 `%20`,否则就直接复制到新的字符串中。最终得到的字符串即为替换后的结果。
C语言:将字符串中的空格替换成 %020 (假定原字符数组能够存放替换后的字符串)。函数只传递一个参数
可以使用以下C语言函数来将字符串中的空格替换成 %020:
```c
void replace_space(char *str)
{
int len = strlen(str);
int space_count = 0;
// 计算字符串中空格的数量
for (int i = 0; i < len; i++) {
if (str[i] == ' ') {
space_count++;
}
}
// 计算替换后的字符串长度
int new_len = len + space_count * 19;
// 从后往前遍历字符串,进行替换操作
for (int i = len - 1; i >= 0; i--) {
if (str[i] == ' ') {
// 如果是空格,则替换为 %020
str[new_len - 1] = '0';
str[new_len - 2] = '2';
str[new_len - 3] = '%';
new_len -= 3;
} else {
// 如果不是空格,则直接复制
str[new_len - 1] = str[i];
new_len--;
}
}
}
```
这个函数会先计算字符串中空格的数量,然后计算替换后的字符串长度,并从后往前遍历字符串进行替换操作。最终将替换结果存放在原字符数组中。
相关推荐
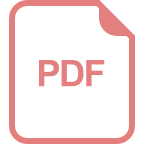
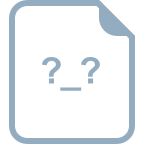
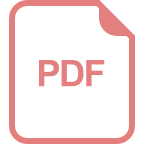
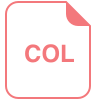
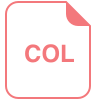
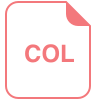
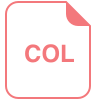
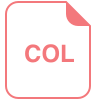







