android sqlite 存图片,Android sqlite 存取图片
时间: 2023-09-08 16:17:31 浏览: 205
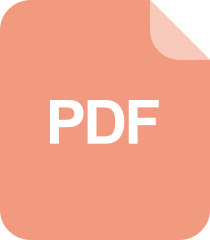
Android数据库SD卡创建和图片存取操作
在 Android SQLite 中存储图片通常有两种方法:
1. 将图片转换为二进制数据并存储在 SQLite 数据库中。
2. 将图片存储在设备的存储空间中,并在 SQLite 数据库中存储图片的路径。
以下是第一种方法的代码示例:
1. 将图片转换为字节数组
```
ByteArrayOutputStream stream = new ByteArrayOutputStream();
Bitmap bitmap = BitmapFactory.decodeResource(context.getResources(), R.drawable.image);
bitmap.compress(Bitmap.CompressFormat.PNG, 100, stream);
byte[] byteArray = stream.toByteArray();
```
2. 将字节数组存储在 SQLite 数据库中
```
SQLiteDatabase db = this.getWritableDatabase();
ContentValues cv = new ContentValues();
cv.put(COLUMN_NAME, "image_name");
cv.put(COLUMN_IMAGE, byteArray);
db.insert(TABLE_NAME, null, cv);
```
3. 从 SQLite 数据库中检索图像并显示
```
SQLiteDatabase db = this.getReadableDatabase();
String[] columns = {COLUMN_IMAGE};
String selection = COLUMN_NAME + "=?";
String[] selectionArgs = {"image_name"};
Cursor cursor = db.query(TABLE_NAME, columns, selection, selectionArgs, null, null, null);
if(cursor.moveToFirst()){
byte[] byteArray = cursor.getBlob(0);
Bitmap bitmap = BitmapFactory.decodeByteArray(byteArray, 0, byteArray.length);
imageView.setImageBitmap(bitmap);
}
cursor.close();
db.close();
```
以下是第二种方法的代码示例:
1. 将图片存储在设备的存储空间中
```
String imagePath = Environment.getExternalStorageDirectory() + "/image.jpg";
Bitmap bitmap = BitmapFactory.decodeResource(context.getResources(), R.drawable.image);
FileOutputStream outputStream = new FileOutputStream(imagePath);
bitmap.compress(Bitmap.CompressFormat.PNG, 100, outputStream);
outputStream.flush();
outputStream.close();
```
2. 将图片的路径存储在 SQLite 数据库中
```
SQLiteDatabase db = this.getWritableDatabase();
ContentValues cv = new ContentValues();
cv.put(COLUMN_NAME, "image_name");
cv.put(COLUMN_IMAGE_PATH, imagePath);
db.insert(TABLE_NAME, null, cv);
```
3. 从 SQLite 数据库中检索图像路径并显示
```
SQLiteDatabase db = this.getReadableDatabase();
String[] columns = {COLUMN_IMAGE_PATH};
String selection = COLUMN_NAME + "=?";
String[] selectionArgs = {"image_name"};
Cursor cursor = db.query(TABLE_NAME, columns, selection, selectionArgs, null, null, null);
if(cursor.moveToFirst()){
String imagePath = cursor.getString(0);
Bitmap bitmap = BitmapFactory.decodeFile(imagePath);
imageView.setImageBitmap(bitmap);
}
cursor.close();
db.close();
```
请注意,第二种方法可能更具可扩展性,因为它允许您在不更改数据库结构的情况下更改图像。但是,它的缺点是需要存储设备上的额外空间,并且需要管理图片文件的名称和位置等细节。
阅读全文
相关推荐
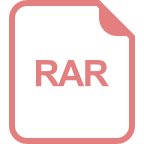
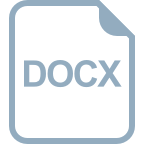









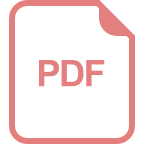
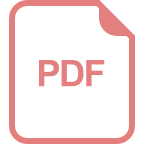
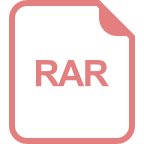
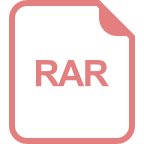
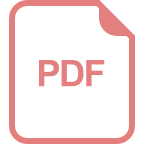
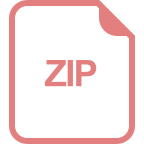