在Web API项目中,用Swagger描述API接口的例子?
时间: 2023-06-19 18:09:55 浏览: 102
以下是一个使用Swagger描述API接口的Web API项目的示例:
```csharp
using System.Collections.Generic;
using Microsoft.AspNetCore.Mvc;
namespace MyWebApi.Controllers
{
[ApiController]
[Route("[controller]")]
public class UsersController : ControllerBase
{
/// <summary>
/// 获取所有用户
/// </summary>
/// <returns>用户列表</returns>
[HttpGet]
[ProducesResponseType(typeof(IEnumerable<User>), 200)]
public IActionResult GetAllUsers()
{
return Ok(new List<User>
{
new User { Id = 1, Name = "Alice" },
new User { Id = 2, Name = "Bob" }
});
}
/// <summary>
/// 获取指定用户
/// </summary>
/// <param name="id">用户ID</param>
/// <returns>用户信息</returns>
[HttpGet("{id}")]
[ProducesResponseType(typeof(User), 200)]
[ProducesResponseType(404)]
public IActionResult GetUser(int id)
{
var user = new User { Id = id, Name = "Alice" };
if (user == null)
{
return NotFound();
}
return Ok(user);
}
/// <summary>
/// 添加用户
/// </summary>
/// <param name="user">用户信息</param>
/// <returns>添加结果</returns>
[HttpPost]
[ProducesResponseType(typeof(User), 201)]
[ProducesResponseType(400)]
public IActionResult AddUser(User user)
{
if (user == null)
{
return BadRequest();
}
user.Id = 3; // 模拟分配ID
return CreatedAtAction(nameof(GetUser), new { id = user.Id }, user);
}
/// <summary>
/// 更新用户
/// </summary>
/// <param name="id">用户ID</param>
/// <param name="user">用户信息</param>
/// <returns>更新结果</returns>
[HttpPut("{id}")]
[ProducesResponseType(204)]
[ProducesResponseType(400)]
[ProducesResponseType(404)]
public IActionResult UpdateUser(int id, User user)
{
if (user == null || user.Id != id)
{
return BadRequest();
}
// 更新用户信息
return NoContent();
}
/// <summary>
/// 删除用户
/// </summary>
/// <param name="id">用户ID</param>
/// <returns>删除结果</returns>
[HttpDelete("{id}")]
[ProducesResponseType(204)]
[ProducesResponseType(404)]
public IActionResult DeleteUser(int id)
{
// 删除用户信息
return NoContent();
}
}
public class User
{
public int Id { get; set; }
public string Name { get; set; }
}
}
```
在上面的示例中,我们使用了 `[ApiController]` 和 `[Route]` 特性来定义控制器和路由。其中,`[ApiController]` 告诉 ASP.NET Core 这是一个 Web API 控制器,并使用默认的行为,例如自动模型绑定和验证。`[Route]` 特性指定了控制器的路由前缀。
每个操作方法都有一个 HTTP 动词特性,例如 `[HttpGet]`,指定了该方法对应的 HTTP GET 请求。我们还使用了`[ProducesResponseType]` 特性,用于指定操作方法的返回类型及其 HTTP 状态码。这些信息可以帮助 Swagger 自动生成 API 文档。
在上面的示例中,我们使用了标准的 HTTP 状态码和响应模型。但是,你也可以使用自定义的状态码和响应模型,只需在`[ProducesResponseType]` 特性中指定即可。
当你启用 Swagger 后,你可以在浏览器中访问 `http://localhost:<port>/swagger` 查看自动生成的 API 文档。
相关推荐
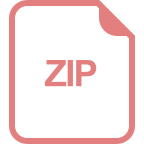
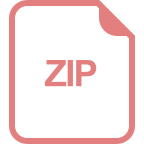









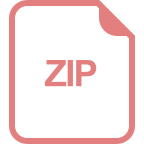
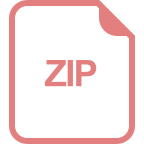
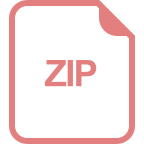
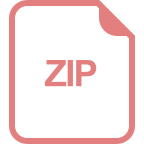
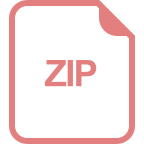
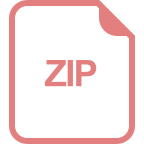