前端vue实现时间刻度尺
时间: 2023-09-06 16:11:03 浏览: 102
时间刻度尺可以使用 Vue.js 的自定义组件来实现。下面是一个简单的实现示例:
```vue
<template>
<div class="time-scale">
<div class="time-scale__ruler">
<div class="time-scale__ruler-line" v-for="i in rulerCount" :key="i" :style="{ left: i * rulerWidth + 'px' }"></div>
</div>
<div class="time-scale__labels">
<div class="time-scale__label" v-for="label in labels" :key="label" :style="{ left: label.position + 'px' }">{{ label.text }}</div>
</div>
</div>
</template>
<script>
export default {
props: {
startTime: {
type: Date,
required: true
},
endTime: {
type: Date,
required: true
},
labelInterval: {
type: Number,
default: 1
}
},
computed: {
rulerCount() {
const duration = this.endTime - this.startTime;
const interval = this.labelInterval * 60 * 60 * 1000;
return Math.ceil(duration / interval);
},
rulerWidth() {
return 100 / this.rulerCount;
},
labels() {
const labels = [];
const interval = this.labelInterval * 60 * 60 * 1000;
for (let i = 0; i <= this.rulerCount; i++) {
const time = new Date(this.startTime.getTime() + i * interval);
labels.push({
text: time.toLocaleTimeString([], { hour: '2-digit', minute: '2-digit' }),
position: i * this.rulerWidth
});
}
return labels;
}
}
};
</script>
<style scoped>
.time-scale {
position: relative;
height: 50px;
border: 1px solid #ccc;
}
.time-scale__ruler {
position: absolute;
top: 0;
left: 0;
right: 0;
bottom: 20px;
}
.time-scale__ruler-line {
position: absolute;
top: 0;
bottom: 0;
width: 1px;
background-color: #ccc;
}
.time-scale__labels {
position: absolute;
bottom: 0;
left: 0;
right: 0;
height: 20px;
display: flex;
justify-content: space-between;
}
.time-scale__label {
position: absolute;
bottom: 0;
font-size: 12px;
white-space: nowrap;
}
</style>
```
在上面的示例中,我们定义了一个名为 `TimeScale` 的组件,它接受三个属性:
- `startTime`:起始时间,必需的。
- `endTime`:结束时间,必需的。
- `labelInterval`:标签间隔,以小时为单位,默认为 1。
然后,我们使用计算属性来计算刻度尺中的一些值,例如刻度线的数量、刻度线的宽度和标签的位置和文本。最后,我们在模板中使用 `v-for` 指令来渲染刻度线和标签。
相关推荐
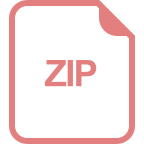
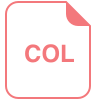
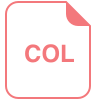
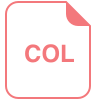
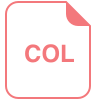
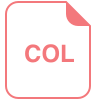









