qt5如何动态添加dll
时间: 2023-12-08 12:04:30 浏览: 31
在 Qt5 中动态添加 dll(动态链接库)可以使用 QLibrary 类。QLibrary 类提供了动态链接库的加载和卸载功能,能够将动态链接库加载到进程中,并在程序运行时使用其中的函数。
以下是动态添加 dll 的基本步骤:
1. 包含 QLibrary 头文件:
```cpp
#include <QLibrary>
```
2. 创建 QLibrary 对象,指定 dll 文件路径:
```cpp
QLibrary myLib("myDll.dll");
```
3. 使用 load() 函数加载 dll:
```cpp
if(myLib.load()){
// dll 加载成功
}else{
// dll 加载失败
}
```
4. 使用 resolve() 函数获取 dll 中的函数指针:
```cpp
// 获取函数指针
typedef void (*MyFunction)();
MyFunction myFunction = (MyFunction)myLib.resolve("myFunction");
if(myFunction){
// 调用函数
myFunction();
}else{
// 获取函数指针失败
}
```
5. 在程序退出前,使用 unload() 函数卸载 dll:
```cpp
myLib.unload();
```
完整示例代码:
```cpp
#include <QLibrary>
#include <QDebug>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 创建 QLibrary 对象,指定 dll 文件路径
QLibrary myLib("myDll.dll");
// 加载 dll
if(myLib.load()){
qDebug() << "dll loaded successfully";
// 获取函数指针
typedef void (*MyFunction)();
MyFunction myFunction = (MyFunction)myLib.resolve("myFunction");
if(myFunction){
// 调用函数
myFunction();
}else{
qDebug() << "get function pointer failed";
}
// 卸载 dll
myLib.unload();
}else{
qDebug() << "dll loaded failed";
}
return a.exec();
}
```
注意:
1. dll 文件必须位于应用程序可执行文件同一目录下或系统路径中。
2. 在使用 resolve() 函数获取函数指针时,需要指定函数的名称,并且需要将函数指针强制转换为正确的类型。
相关推荐
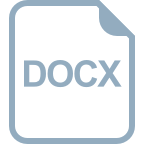














