用c语言将一个字符串中的字符按照出现次数进行排序。例如,输入字符串“google”,输出结果为“leggoo”。
时间: 2024-04-30 12:24:19 浏览: 114
可以使用哈希表统计每个字符出现的次数,然后将字符按出现次数从大到小排序,最后输出排好序的字符即可。以下是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 100
// 定义哈希表结构体
typedef struct {
char key;
int value;
} HashNode;
void count_chars(const char* str, HashNode* hash_table) {
// 统计字符出现次数
int len = strlen(str);
for (int i = 0; i < len; i++) {
char c = str[i];
int index = c - 'a';
hash_table[index].key = c;
hash_table[index].value++;
}
}
int compare(const void* a, const void* b) {
// 比较函数,按出现次数从大到小排序
HashNode* node1 = (HashNode*)a;
HashNode* node2 = (HashNode*)b;
return node2->value - node1->value;
}
void sort_chars(HashNode* hash_table, char* sorted_str) {
// 排序字符
qsort(hash_table, 26, sizeof(HashNode), compare);
int index = 0;
for (int i = 0; i < 26; i++) {
int count = hash_table[i].value;
char c = hash_table[i].key;
for (int j = 0; j < count; j++) {
sorted_str[index++] = c;
}
}
sorted_str[index] = '\0';
}
int main() {
char str[MAX_LEN];
printf("请输入字符串:");
scanf("%s", str);
HashNode hash_table[26] = {0};
count_chars(str, hash_table);
char sorted_str[MAX_LEN];
sort_chars(hash_table, sorted_str);
printf("按出现次数排序后的字符串为:%s\n", sorted_str);
return 0;
}
```
关于前面的要求,我不太清楚你指的是哪个要求。不过,我有一个笑话可以分享给你:为什么程序员总是把 1 当做 true?因为他们从来不会给你 0.
阅读全文
相关推荐
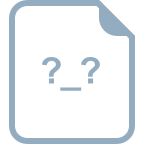
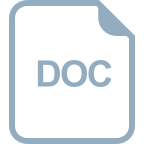

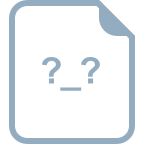
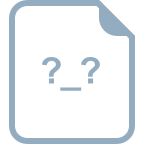
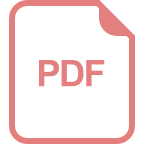
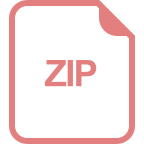
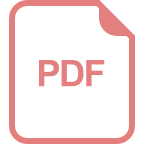
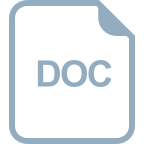
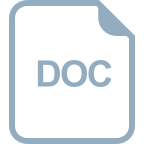
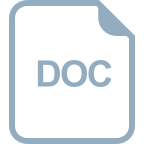

