用import numpy as np from skimage.io import imread from skimage.color import rgb2hsv,hsv2rgb from skimage.exposure import equalize_hist import matplotlib.pyplot as plt plt.rcParams['font.sans-serif'] = ['SimHei'] # 指定默认字体 plt.rcParams['axes.unicode_minus'] = False # 解决保存图像是负号'-'显示为方块的问题 A=np.clip(B, 0, 1.0) # 对数字B的每个元素值进行两头截断,确保输出数组A的元素取值在0~1之间
时间: 2024-02-13 09:04:34 浏览: 167
好的,这段代码的功能是导入必要的Python库,并设置一些绘图常用的参数,以及展示如何使用`numpy.clip()`函数对数组进行截断操作。
代码如下:
```python
import numpy as np
from skimage.io import imread
from skimage.color import rgb2hsv, hsv2rgb
from skimage.exposure import equalize_hist
import matplotlib.pyplot as plt
# 设置绘图参数
plt.rcParams['font.sans-serif'] = ['SimHei'] # 指定默认字体
plt.rcParams['axes.unicode_minus'] = False # 解决保存图像是负号'-'显示为方块的问题
# 对数字B的每个元素值进行两头截断,确保输出数组A的元素取值在0~1之间
A = np.clip(B, 0, 1.0)
```
需要注意的是,上述代码中的`B`是一个数组,可以替换成实际需要处理的数组。此外,`numpy.clip()`函数的第二个参数和第三个参数分别表示截断的下限和上限,可以根据实际情况自行调整。
相关问题
import cv2 as cv from numpy import * import numpy as np from skimage import io import glob
这是一个 Python 的代码段,它导入了以下库:
- cv2:OpenCV 库,用于处理图像和视频;
- numpy:Python 的科学计算库,提供了多维数组对象和各种派生对象(如掩码数组、矩阵等)以及用于数组操作的函数;
- skimage:基于 Python 的图像处理库,提供了大量的图像处理功能;
- glob:Python 的标准库之一,用于查找符合特定规则的文件路径名。
此外,代码中还定义了一个名为 io 的函数,但是在代码中并没有使用到。
import numpy as npimport cv2import osimport tqdmimport argparsefrom skimage.draw import polygonimport random
这段代码导入了几个常用的Python库,用于图像处理和操作:
1. `numpy` 是数值计算的基础库,提供了大量的数学和逻辑运算功能。
2. `cv2`(OpenCV)是一个广泛使用的计算机视觉库,常用于图像读取、处理和分析。
3. `os` 提供操作系统相关的功能,如文件路径操作、目录管理等。
4. `tqdm` 是一个进度条库,用于显示长时间运行任务的进度。
5. `argparse` 是解析命令行参数的标准库,方便处理用户输入。
6. `skimage.draw.polygon` 应该是`scikit-image`的一部分,用于绘制二维图形,特别是与图像处理相关的几何形状。
7. `random` 包含各种随机数生成器,常用于模拟和概率算法。
这些库结合在一起可以实现图像处理流程中的各个环节,例如加载图像、预处理、特征提取、绘制结果或执行需要大量迭代的操作时展示进度。
下面是一些可能的用法示例:
```python
# 加载图片
img_path = 'path/to/image.jpg'
img = cv2.imread(img_path)
# 显示图片并添加进度条
for i in tqdm(range(100)):
# 对图片进行某种操作...
pass
# 使用numpy进行数组操作
arr = np.random.rand(100, 100)
max_value = arr.max()
# 绘制多边形
points = [(x, y) for x, y in zip(random.sample(range(100), 5), random.sample(range(100), 5))]
polygon_img = np.zeros((100, 100))
polygon(polygon_img, points, color=(255, 0, 0))
# 解析命令行参数
parser = argparse.ArgumentParser()
parser.add_argument('--image', help='Path to the image')
args = parser.parse_args()
if args.image:
img = cv2.imread(args.image)
```
阅读全文
相关推荐
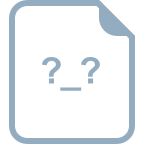
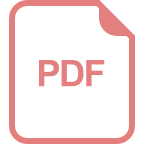













