针对微博内容怎么做预处理,并且保存到新的csv文件中
时间: 2024-03-14 15:45:33 浏览: 15
针对微博内容的预处理一般包括以下几个步骤:
1. 去除 HTML 标签和表情符号。
2. 去除网址和@用户。
3. 分词,去除停用词和标点符号。
4. 进行词性标注和命名实体识别(可选)。
下面是一份示例代码,它使用了 jieba 库进行分词,使用了 pandas 库读写 csv 文件:
```python
import re
import jieba
import pandas as pd
# 读取微博数据
df = pd.read_csv('weibo.csv')
# 去除 HTML 标签和表情符号
def clean_text(text):
# 去除 HTML 标签
text = re.sub('<[^>]+>', '', text)
# 去除表情符号
text = re.sub('\[.*?\]', '', text)
return text
# 去除网址和@用户
def remove_url_and_at(text):
# 去除网址
text = re.sub('https?://[^\s]+', '', text)
# 去除@用户
text = re.sub('@[^\s]+', '', text)
return text
# 分词,去除停用词和标点符号
def tokenize(text):
# 分词
words = jieba.cut(text)
# 去除停用词和标点符号
stopwords = set(['的', '了', '是', '我', '你', '他', '她', '我们', '你们', '他们'])
words = [word.strip() for word in words if word.strip() not in stopwords and re.match(r'^[\w\u4e00-\u9fa5]+$', word)]
return words
# 对每条微博进行处理
for i, row in df.iterrows():
text = row['text']
# 去除 HTML 标签和表情符号
text = clean_text(text)
# 去除网址和@用户
text = remove_url_and_at(text)
# 分词,去除停用词和标点符号
words = tokenize(text)
# 将处理后的文本保存到新列中
df.at[i, 'processed_text'] = ' '.join(words)
# 将处理后的数据保存到新的 csv 文件中
df.to_csv('weibo_processed.csv', index=False, encoding='utf-8-sig')
```
上面的代码中,我们首先读取了微博数据文件 `weibo.csv`,然后定义了三个函数 `clean_text`、`remove_url_and_at` 和 `tokenize`,它们分别用于去除 HTML 标签和表情符号、去除网址和@用户以及分词、去除停用词和标点符号。然后,我们对每条微博进行处理,并将处理后的文本保存到新列 `processed_text` 中。最后,我们使用 pandas 库将处理后的数据保存到新的 csv 文件 `weibo_processed.csv` 中。
相关推荐
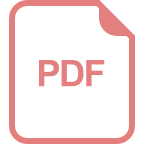














