java 两个 list<map>个数不同 找到map 中不同的key 和value 示例
时间: 2023-05-24 07:03:18 浏览: 233
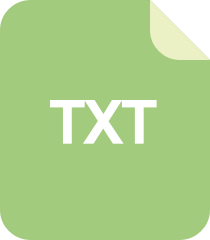
封装ResultSet结果集
假设要找到两个 List<Map<String, Object>> 中不同的 key 和 value:
```java
List<Map<String, Object>> list1 = new ArrayList<>();
List<Map<String, Object>> list2 = new ArrayList<>();
// 假设 list1 包含 3 个 Map,而 list2 包含 4 个 Map
list1.add(new HashMap<String, Object>() {{
put("key1", 1);
put("key2", "value2");
put("key3", true);
}});
list1.add(new HashMap<String, Object>() {{
put("key1", 2);
put("key2", "value2");
put("key4", "value4");
}});
list1.add(new HashMap<String, Object>() {{
put("key1", 3);
put("key2", "value2");
put("key5", true);
}});
list2.add(new HashMap<String, Object>() {{
put("key1", 1);
put("key2", "value2");
put("key3", true);
}});
list2.add(new HashMap<String, Object>() {{
put("key1", 2);
put("key2", "value2");
put("key4", "different_value"); // 不同
}});
list2.add(new HashMap<String, Object>() {{
put("key1", 3);
put("key2", "value2");
put("key5", true);
}});
list2.add(new HashMap<String, Object>() {{
put("key1", 4);
put("key2", "value2");
put("key6", false); // 不同
}});
// 用一个 HashSet 保存所有的 key-value 对
Set<Map.Entry<String, Object>> entries = new HashSet<>();
for (Map<String, Object> map : list1) {
entries.addAll(map.entrySet());
}
for (Map<String, Object> map : list2) {
entries.addAll(map.entrySet());
}
// 遍历所有的 key-value 对,找到不同的对
for (Map.Entry<String, Object> entry : entries) {
String key = entry.getKey();
Object value = entry.getValue();
boolean foundInList1 = false;
boolean foundInList2 = false;
for (Map<String, Object> map : list1) {
if (map.containsKey(key) && map.get(key).equals(value)) {
foundInList1 = true;
break;
}
}
for (Map<String, Object> map : list2) {
if (map.containsKey(key) && map.get(key).equals(value)) {
foundInList2 = true;
break;
}
}
if (foundInList1 != foundInList2) {
System.out.println("Different key-value pair: " + key + "=" + value);
}
}
```
上述代码遍历所有的 key-value 对,然后分别在两个 List 中查找是否存在这个 key-value 对。如果两个 List 中某一个存在这个 key-value 对而另一个不存在,则认为这个 key-value 对是不同的。输出所有不同的 key-value 对。
阅读全文
相关推荐
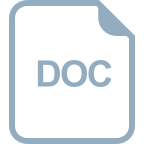
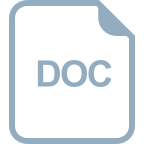





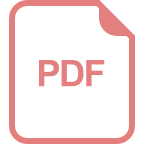









