.net core 连接SQL server数据库的带代码是什么 写在哪 并且dbhelp的代码
时间: 2024-01-24 13:17:28 浏览: 83
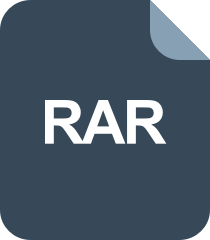
通过代码连接SQL Server数据库
在.NET Core中连接SQL Server数据库,可以使用ADO.NET提供的System.Data.SqlClient命名空间中的类来实现。以下是一个简单的示例:
```csharp
using System.Data.SqlClient;
string connectionString = "Data Source=myServerAddress;Initial Catalog=myDataBase;User ID=myUsername;Password=myPassword;";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
// 执行查询或操作
}
```
其中,`connectionString`是连接字符串,包含了需要连接的SQL Server的地址、数据库名称、用户名和密码等信息。使用`SqlConnection`类打开数据库连接,然后就可以执行查询或操作了。
如果想要使用dbhelper,可以使用Dapper库来简化数据库操作。以下是一个使用Dapper的示例:
```csharp
using Dapper;
using System.Collections.Generic;
using System.Data.SqlClient;
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
}
public class CustomerRepository
{
private readonly string _connectionString;
public CustomerRepository(string connectionString)
{
_connectionString = connectionString;
}
public IEnumerable<Customer> GetAll()
{
using (var connection = new SqlConnection(_connectionString))
{
connection.Open();
return connection.Query<Customer>("SELECT * FROM Customers");
}
}
public void Add(Customer customer)
{
using (var connection = new SqlConnection(_connectionString))
{
connection.Open();
connection.Execute("INSERT INTO Customers (Name, Email) VALUES (@Name, @Email)", customer);
}
}
}
```
在上面的示例中,`Customer`类表示数据库中的一条记录,`CustomerRepository`类封装了对`Customers`表的操作。使用`Dapper`库可以很方便地执行查询和修改操作,不用手动写SQL语句。
阅读全文
相关推荐
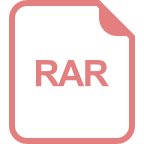





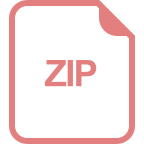
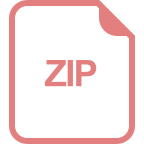
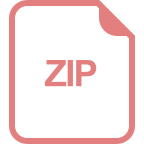
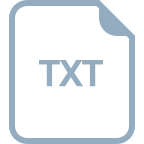
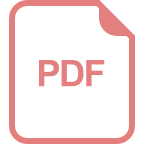
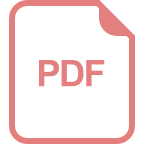
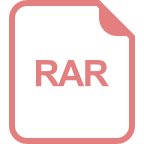
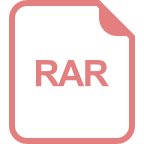