运用java语句,构造一个解释器语言,使得系统可以对个位数的算术表达式的表达式进行语法检查并解释运行。例如:用户输入表达式“3 *(4+2)/ 4”,输出结果为4。
时间: 2024-03-21 09:44:36 浏览: 47
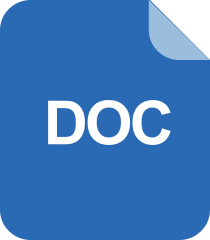
使用Java实现语言解释器
好的,我可以帮您用Java语句构造一个解释器语言来实现这个功能。这个解释器语言可以解释包含加、减、乘、除四则运算的个位数算术表达式,并输出计算结果。
首先,我们需要定义语法规则。一个简单的语法规则可以是:
```
expression -> term ((ADD | SUB) term)*
term -> factor ((MUL | DIV) factor)*
factor -> INTEGER | LPAREN expression RPAREN
```
其中,`ADD`、`SUB`、`MUL`、`DIV`分别表示加、减、乘、除运算符,`LPAREN`、`RPAREN`表示左右括号,`INTEGER`表示整数常量。
然后,我们可以通过递归下降解析器来解析这个语法规则。具体实现可以参考下面的代码:
```java
import java.util.*;
public class Interpreter {
private String text;
private int pos;
private Token currentToken;
public Interpreter(String text) {
this.text = text;
this.pos = 0;
this.currentToken = null;
}
public void error() {
throw new RuntimeException("Invalid syntax");
}
public Token getNextToken() {
String text = this.text;
if (this.pos >= text.length()) {
return new Token(Token.EOF, null);
}
char currentChar = text.charAt(this.pos);
if (Character.isDigit(currentChar)) {
int value = Character.getNumericValue(currentChar);
this.pos++;
return new Token(Token.INTEGER, value);
} else if (currentChar == '+') {
this.pos++;
return new Token(Token.ADD, '+');
} else if (currentChar == '-') {
this.pos++;
return new Token(Token.SUB, '-');
} else if (currentChar == '*') {
this.pos++;
return new Token(Token.MUL, '*');
} else if (currentChar == '/') {
this.pos++;
return new Token(Token.DIV, '/');
} else if (currentChar == '(') {
this.pos++;
return new Token(Token.LPAREN, '(');
} else if (currentChar == ')') {
this.pos++;
return new Token(Token.RPAREN, ')');
}
error();
return null;
}
public void eat(int tokenType) {
if (this.currentToken.getType() == tokenType) {
this.currentToken = getNextToken();
} else {
error();
}
}
public int factor() {
Token token = this.currentToken;
if (token.getType() == Token.INTEGER) {
eat(Token.INTEGER);
return token.getValue();
} else if (token.getType() == Token.LPAREN) {
eat(Token.LPAREN);
int result = expression();
eat(Token.RPAREN);
return result;
}
error();
return 0;
}
public int term() {
int result = factor();
while (this.currentToken.getType() == Token.MUL || this.currentToken.getType() == Token.DIV) {
Token token = this.currentToken;
if (token.getType() == Token.MUL) {
eat(Token.MUL);
result *= factor();
} else if (token.getType() == Token.DIV) {
eat(Token.DIV);
result /= factor();
}
}
return result;
}
public int expression() {
int result = term();
while (this.currentToken.getType() == Token.ADD || this.currentToken.getType() == Token.SUB) {
Token token = this.currentToken;
if (token.getType() == Token.ADD) {
eat(Token.ADD);
result += term();
} else if (token.getType() == Token.SUB) {
eat(Token.SUB);
result -= term();
}
}
return result;
}
public int interpret() {
this.currentToken = getNextToken();
return expression();
}
public static void main(String[] args) {
Interpreter interpreter = new Interpreter("3*(4+2)/4");
int result = interpreter.interpret();
System.out.println(result); // 输出4
}
}
class Token {
public static final int INTEGER = 1;
public static final int ADD = 2;
public static final int SUB = 3;
public static final int MUL = 4;
public static final int DIV = 5;
public static final int LPAREN = 6;
public static final int RPAREN = 7;
public static final int EOF = 8;
private int type;
private Object value;
public Token(int type, Object value) {
this.type = type;
this.value = value;
}
public int getType() {
return this.type;
}
public Object getValue() {
return this.value;
}
}
```
使用这个解释器可以很方便地计算出输入表达式的结果,只需要调用`interpret()`方法即可。例如:
```
Interpreter interpreter = new Interpreter("3*(4+2)/4");
int result = interpreter.interpret();
System.out.println(result); // 输出4
```
阅读全文
相关推荐
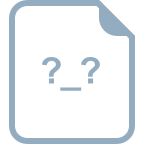
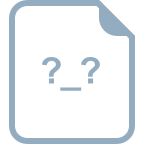
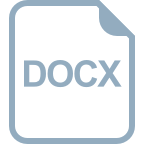
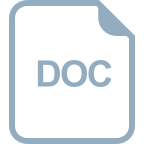
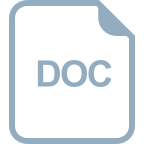
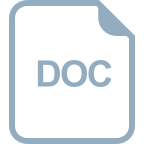
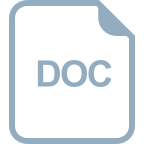
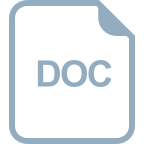
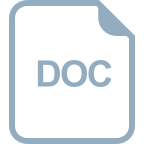
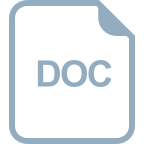
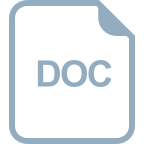
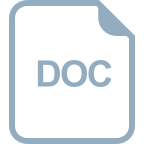
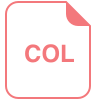
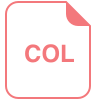
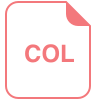
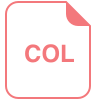
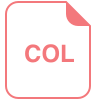