python 实现接口文档导出和下载功能
时间: 2024-05-10 07:19:29 浏览: 141
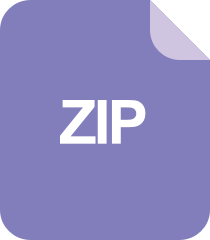
python 下载文件
Python 可以使用第三方库 `flasgger` 来实现接口文档的自动生成和下载功能。`flasgger` 通过解析 API 的注释来自动生成接口文档,并提供了下载文档的功能。
安装 `flasgger`:
```
pip install flasgger
```
在 Flask 应用中,使用 `flasgger` 的 `Swagger` 类来配置接口文档:
```python
from flask import Flask
from flasgger import Swagger
app = Flask(__name__)
swagger = Swagger(app)
@app.route('/hello')
def hello():
"""
This is an example endpoint that returns a greeting.
---
responses:
200:
description: A greeting message
"""
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
```
在上面的例子中,`hello` 函数的注释被解析为接口文档中的描述。`responses` 字段指定了响应的格式。运行应用后,在浏览器中访问 `http://localhost:5000/apidocs/` 就可以查看接口文档了。
要实现接口文档的下载功能,可以在应用中添加一个路由,在该路由中使用 `flasgger` 的 `swag_from` 装饰器来加载接口文档,并将其保存为文件:
```python
from flask import Flask, send_file
from flasgger import Swagger, swag_from
app = Flask(__name__)
swagger = Swagger(app)
@app.route('/download')
@swag_from('swagger.yaml')
def download():
"""
Download the API documentation in YAML format.
"""
with open('swagger.yaml', 'r') as f:
data = f.read()
return send_file(data, attachment_filename='swagger.yaml', as_attachment=True)
@app.route('/hello')
def hello():
"""
This is an example endpoint that returns a greeting.
---
responses:
200:
description: A greeting message
"""
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
```
在上面的例子中,`download` 函数使用 `swag_from` 装饰器来加载接口文档。`send_file` 函数将文件作为响应发送给客户端。`attachment_filename` 参数指定下载文件的名称,`as_attachment` 参数指定将文件作为附件发送,而不是内联显示。
要使用 `flasgger` 自动生成的接口文档,需要在应用根目录下创建一个名为 `swagger.yaml` 的文件,并将以下内容复制到该文件中:
```yaml
swagger: '2.0'
info:
version: '1.0'
title: Sample API
description: Sample API for demonstration purposes only.
paths: {}
```
运行应用后,在浏览器中访问 `http://localhost:5000/download` 就可以下载接口文档了。
阅读全文
相关推荐
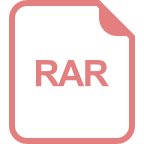
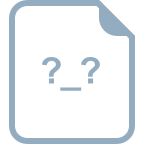
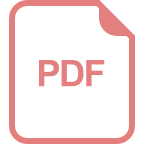
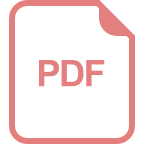
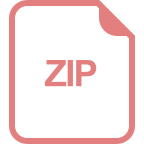
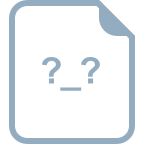
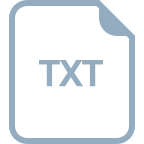
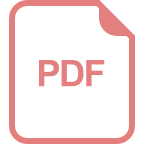
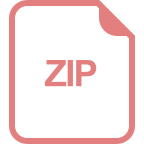
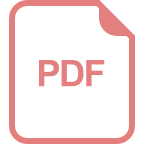
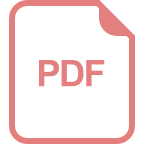
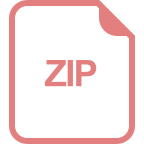
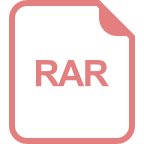
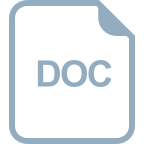
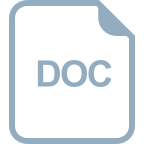
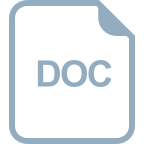
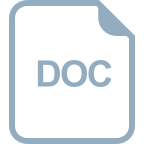