qt create debug日志路径
时间: 2023-09-01 15:13:34 浏览: 106
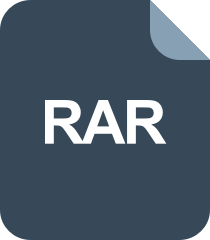
Qt实现简单的qDebug输出到日志文件

在 Qt 中,您可以通过以下方法设置创建调试日志的路径:
1. 在 main 函数中设置日志路径:
```
#include <QCoreApplication>
#include <QLoggingCategory>
#include <QDebug>
#include <QFile>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 设置日志路径
QString logPath = "debug.log";
QFile file(logPath);
file.open(QIODevice::WriteOnly | QIODevice::Append);
qInstallMessageHandler([](QtMsgType type, const QMessageLogContext &context, const QString &msg)
{
QTextStream out(&file);
switch (type)
{
case QtDebugMsg:
out << "Debug: " << msg << endl;
break;
case QtWarningMsg:
out << "Warning: " << msg << endl;
break;
case QtCriticalMsg:
out << "Critical: " << msg << endl;
break;
case QtFatalMsg:
out << "Fatal: " << msg << endl;
abort();
}
});
qInfo() << "Hello, world!";
return a.exec();
}
```
2. 使用 qInstallMessageHandler 函数设置日志路径:
```
#include <QCoreApplication>
#include <QLoggingCategory>
#include <QDebug>
#include <QFile>
// 日志处理函数
void messageHandler(QtMsgType type, const QMessageLogContext &context, const QString &msg)
{
static QFile file("debug.log");
if (!file.isOpen())
{
file.open(QIODevice::WriteOnly | QIODevice::Append);
}
QTextStream out(&file);
switch (type)
{
case QtDebugMsg:
out << "Debug: " << msg << endl;
break;
case QtWarningMsg:
out << "Warning: " << msg << endl;
break;
case QtCriticalMsg:
out << "Critical: " << msg << endl;
break;
case QtFatalMsg:
out << "Fatal: " << msg << endl;
abort();
}
}
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 设置日志处理函数
qInstallMessageHandler(messageHandler);
qInfo() << "Hello, world!";
return a.exec();
}
```
以上两种方法都会在程序运行时创建一个名为 debug.log 的文件,并将日志记录到该文件中。您可以将文件名更改为您想要的任何名称和路径。
阅读全文
相关推荐
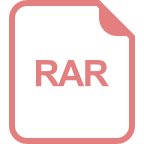
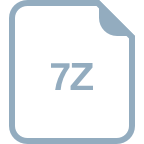

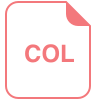
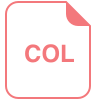

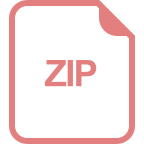
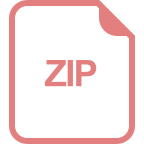
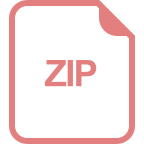
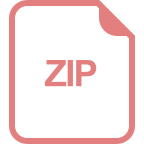
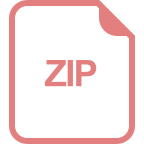
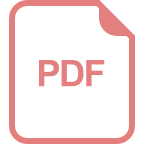
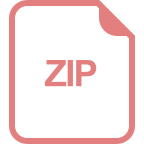
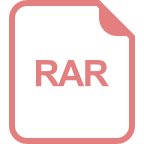
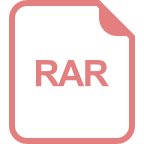
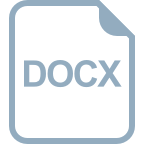