java smtp_java发送邮箱(SMTP协议)
时间: 2023-08-11 17:12:27 浏览: 208
Java中可以使用JavaMail API来发送邮件,其中SMTP协议是最常用的邮件发送协议之一。下面是一个简单的示例代码:
```java
import java.util.Properties;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
public class SMTPJava {
public static void main(String[] args) {
final String username = "your_email@example.com";
final String password = "your_password";
Properties props = new Properties();
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", "smtp.example.com");
props.put("mail.smtp.port", "587");
Session session = Session.getInstance(props,
new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
try {
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress("from@example.com"));
message.setRecipients(Message.RecipientType.TO,
InternetAddress.parse("to@example.com"));
message.setSubject("Testing SMTP Java");
message.setText("Hello, this is a test email from SMTP Java");
Transport.send(message);
System.out.println("Mail sent successfully");
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
}
```
其中,需要修改的参数包括:
- `username`和`password`:你的邮箱账号和密码;
- `props.put("mail.smtp.host", "smtp.example.com");`:将`smtp.example.com`替换为你的邮箱提供商的SMTP服务器地址;
- `message.setFrom(new InternetAddress("from@example.com"));`和`InternetAddress.parse("to@example.com")`:将`from@example.com`和`to@example.com`替换为你的发件人和收件人的邮箱地址。
需要注意的是,有些邮箱提供商可能需要设置授权码(例如Gmail),可以在邮箱设置中找到授权码,并将其替换为密码。
阅读全文
相关推荐
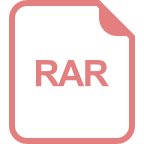
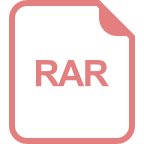
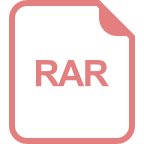
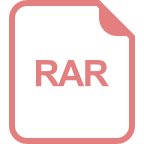
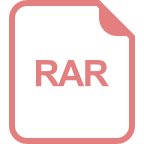
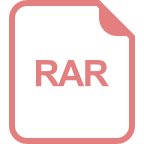
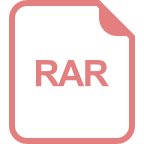
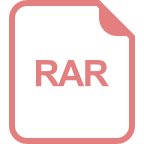
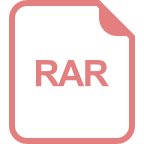
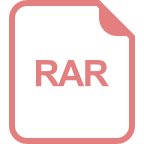
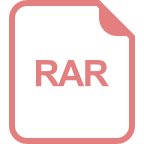
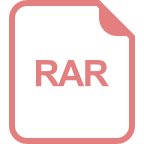
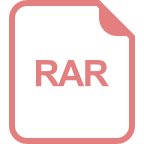
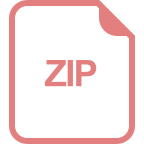
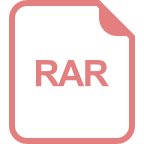