pygame制作范围炮弹
时间: 2023-10-15 20:05:13 浏览: 81
好的,制作范围炮弹的游戏需要以下步骤:
1. 导入pygame模块并初始化
```
import pygame
pygame.init()
```
2. 创建游戏窗口
```
screen = pygame.display.set_mode((width, height))
```
3. 定义颜色常量
```
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
```
4. 定义炮弹类和范围炮弹类
```
class Bullet(pygame.sprite.Sprite):
def __init__(self, x, y, color):
super().__init__()
self.image = pygame.Surface([10, 10])
self.image.fill(color)
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
self.speed = -10
def update(self):
self.rect.y += self.speed
if self.rect.bottom < 0:
self.kill()
class RangeBullet(pygame.sprite.Sprite):
def __init__(self, x, y, color):
super().__init__()
self.image = pygame.Surface([30, 30])
self.image.fill(color)
self.rect = self.image.get_rect()
self.rect.x = x - 10
self.rect.y = y - 10
self.damage = 10
def update(self):
pass
```
5. 创建炮弹和范围炮弹的精灵组
```
bullet_list = pygame.sprite.Group()
range_bullet_list = pygame.sprite.Group()
```
6. 在游戏循环中处理事件和更新炮弹
```
while not done:
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
elif event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1:
bullet = Bullet(player.rect.centerx, player.rect.top, BLUE)
bullet_list.add(bullet)
elif event.button == 3:
range_bullet = RangeBullet(player.rect.centerx, player.rect.centery, RED)
range_bullet_list.add(range_bullet)
bullet_list.update()
range_bullet_list.update()
```
7. 在游戏循环中绘制炮弹
```
screen.fill(WHITE)
bullet_list.draw(screen)
range_bullet_list.draw(screen)
pygame.display.flip()
```
完整代码如下:
阅读全文
相关推荐
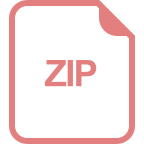
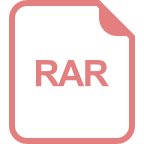















