ssm上传下载 增删改查
时间: 2023-10-11 17:13:42 浏览: 40
SSM是指Spring+SpringMVC+MyBatis,是一个JavaWeb开发框架。下面我将为您介绍SSM框架中的文件上传下载和增删改查操作。
文件上传下载:
1.上传文件
(1)在SpringMVC的配置文件中添加multipartResolver
```
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"/>
```
(2)在Controller中添加上传文件处理方法
```
@RequestMapping(value = "/upload", method = RequestMethod.POST)
public String upload(@RequestParam("file") MultipartFile file, HttpServletRequest request) {
if (!file.isEmpty()) {
try {
String fileName = file.getOriginalFilename();
String filePath = request.getSession().getServletContext().getRealPath("/") + "upload/";
File dest = new File(filePath + fileName);
if (!dest.getParentFile().exists()) {
dest.getParentFile().mkdirs();
}
file.transferTo(dest);
return "success";
} catch (Exception e) {
e.printStackTrace();
}
}
return "error";
}
```
2.下载文件
(1)在Controller中添加下载文件处理方法
```
@RequestMapping(value = "/download", method = RequestMethod.GET)
public void download(HttpServletRequest request, HttpServletResponse response) {
String fileName = "xxx.jpg"; //文件名
String filePath = request.getSession().getServletContext().getRealPath("/") + "upload/";
File file = new File(filePath + fileName);
if (file.exists()) {
response.setContentType("application/force-download");
response.addHeader("Content-Disposition", "attachment;fileName=" + fileName);
byte[] buffer = new byte[1024];
FileInputStream fis = null;
BufferedInputStream bis = null;
try {
fis = new FileInputStream(file);
bis = new BufferedInputStream(fis);
OutputStream os = response.getOutputStream();
int i = bis.read(buffer);
while (i != -1) {
os.write(buffer, 0, i);
i = bis.read(buffer);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (bis != null) {
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fis != null) {
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
```
增删改查:
1.增加数据
(1)在Mapper中添加insert方法
```
public void insert(User user);
```
(2)在Service中添加insert方法
```
public void insert(User user) {
userMapper.insert(user);
}
```
(3)在Controller中添加insert处理方法
```
@RequestMapping(value = "/insert", method = RequestMethod.POST)
public String insert(User user) {
userService.insert(user);
return "success";
}
```
2.删除数据
(1)在Mapper中添加delete方法
```
public void delete(int id);
```
(2)在Service中添加delete方法
```
public void delete(int id) {
userMapper.delete(id);
}
```
(3)在Controller中添加delete处理方法
```
@RequestMapping(value = "/delete", method = RequestMethod.GET)
public String delete(int id) {
userService.delete(id);
return "success";
}
```
3.修改数据
(1)在Mapper中添加update方法
```
public void update(User user);
```
(2)在Service中添加update方法
```
public void update(User user) {
userMapper.update(user);
}
```
(3)在Controller中添加update处理方法
```
@RequestMapping(value = "/update", method = RequestMethod.POST)
public String update(User user) {
userService.update(user);
return "success";
}
```
4.查询数据
(1)在Mapper中添加select方法
```
public List<User> selectAll();
```
(2)在Service中添加selectAll方法
```
public List<User> selectAll() {
return userMapper.selectAll();
}
```
(3)在Controller中添加selectAll处理方法
```
@RequestMapping(value = "/selectAll", method = RequestMethod.GET)
public String selectAll(Model model) {
List<User> userList = userService.selectAll();
model.addAttribute("userList", userList);
return "userList";
}
```
PS:以上代码仅供参考,具体实现需要根据具体业务需求进行修改。
相关推荐
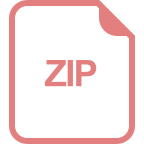












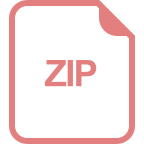