用 pandas numpy matplotlib 爬虫对网易云音乐年度热播榜进行数据分析
时间: 2023-11-17 12:04:36 浏览: 89
首先,我们需要使用爬虫将网易云音乐的年度热播榜上的歌曲信息爬取下来。可以使用 Python 的 requests 和 BeautifulSoup 库来实现。
```python
import requests
from bs4 import BeautifulSoup
url = "https://music.163.com/discover/toplist?id=3778678"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"
}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, "html.parser")
# 获取歌曲列表
song_list = soup.select("ul[class=f-hide] > li > a")
```
接着,我们可以使用 pandas 将歌曲信息转换成 DataFrame 格式,并进行数据分析。以下是一个简单的例子:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# 获取歌曲信息
song_name = [song.text for song in song_list]
song_id = [song["href"].split("=")[-1] for song in song_list]
# 将歌曲信息转换成 DataFrame 格式
data = pd.DataFrame({"歌曲名":song_name, "歌曲ID":song_id})
# 数据分析
# 统计歌曲名中出现最多的词语
words = []
for name in data["歌曲名"]:
words.extend(name.split(" "))
word_counts = pd.Series(words).value_counts()
print("出现最多的词语:")
print(word_counts[:10])
# 统计歌曲在热播榜中的排名分布
data["排名"] = np.arange(1, len(data)+1)
rank_counts = data["排名"].value_counts().sort_index()
plt.bar(rank_counts.index, rank_counts.values)
plt.title("排名分布")
plt.xlabel("排名")
plt.ylabel("歌曲数量")
plt.show()
```
这样就可以对网易云音乐年度热播榜进行数据分析了。当然,这只是一个简单的例子,实际上可以进行更多的数据分析,例如分析歌曲的流派、歌曲评分等。
阅读全文
相关推荐





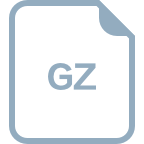






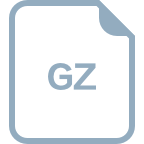




