Java实现分组加密算法 DES
时间: 2023-12-13 09:22:58 浏览: 84
DES(Data Encryption Standard)是一种对称加密算法,它将明文按照一定的规则转换成密文,以保障数据的保密性。下面是使用Java实现DES分组加密算法的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
public class DESExample {
private static final String ALGORITHM = "DES";
private static final String CHARSET = "UTF-8";
public static void main(String[] args) throws Exception {
String message = "Hello, world!";
String password = "12345678";
// 生成密钥
SecretKey secretKey = generateKey(password);
// 加密
byte[] encryptedData = encrypt(message.getBytes(CHARSET), secretKey);
System.out.println("Encrypted data: " + new String(encryptedData, CHARSET));
// 解密
byte[] decryptedData = decrypt(encryptedData, secretKey);
System.out.println("Decrypted data: " + new String(decryptedData, CHARSET));
}
/**
* 生成密钥
*/
public static SecretKey generateKey(String password) throws NoSuchAlgorithmException {
KeyGenerator keyGenerator = KeyGenerator.getInstance(ALGORITHM);
SecureRandom secureRandom = new SecureRandom(password.getBytes());
keyGenerator.init(secureRandom);
return keyGenerator.generateKey();
}
/**
* 加密
*/
public static byte[] encrypt(byte[] data, SecretKey secretKey) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
return cipher.doFinal(data);
}
/**
* 解密
*/
public static byte[] decrypt(byte[] data, SecretKey secretKey) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
return cipher.doFinal(data);
}
}
```
在上面的示例代码中,我们使用了Java自带的`javax.crypto`包中的一些类来实现DES分组加密算法。具体来说,我们使用了`KeyGenerator`类来生成密钥,使用`Cipher`类来进行加密和解密操作。在生成密钥时,我们指定了密钥生成器的算法为DES,并使用了一个随机数生成器来生成密钥。在加密和解密操作时,我们都需要传入一个`SecretKey`对象,这个对象就是我们生成的密钥。
需要注意的是,DES算法的密钥长度为56位,但是Java默认只支持64位的密钥长度。如果想要使用56位的密钥,需要手动将密钥的奇偶校验位去掉,或者使用其他的库来实现。另外,由于DES算法已经被认为不安全,建议使用更加安全的AES算法来进行加密。
阅读全文
相关推荐
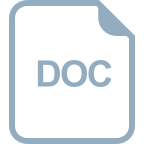
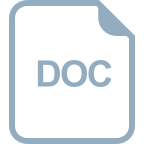
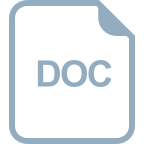
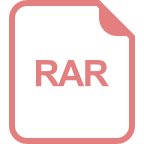

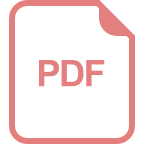
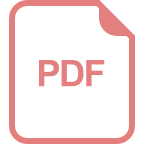
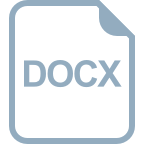
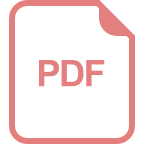
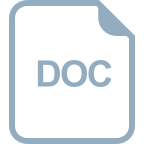
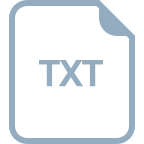
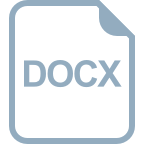
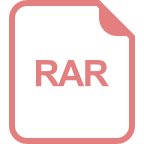
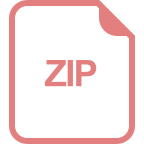
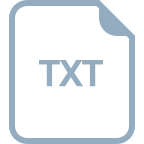
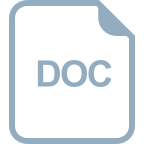
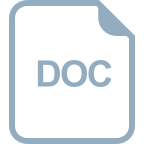
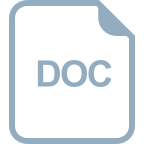