c++输入一串字符串,统计其中字母非字母的数目。
时间: 2024-05-07 10:21:09 浏览: 96
好的,下面是代码实现:
```c
#include <stdio.h>
int main() {
char str[100];
int i, alpha_count = 0, non_alpha_count = 0;
printf("请输入字符串:");
fgets(str, 100, stdin);
for (i = 0; str[i] != '\0'; i++) {
if ((str[i] >= 'a' && str[i] <= 'z') || (str[i] >= 'A' && str[i] <= 'Z')) {
alpha_count++;
} else {
non_alpha_count++;
}
}
printf("字母个数:%d\n非字母个数:%d\n", alpha_count, non_alpha_count);
return 0;
}
```
以上代码通过循环遍历字符串中的每个字符,判断其是否为字母并进行统计,最终输出结果。
相关问题
(C++)现有一个字符串以字符 0 为结尾,其中包含一些空格和回车,现在希望 统计该字符串中空格与回车的数目,并将此字符串中的空格删除、将回车替换为空 格输出。字符串由大小字母、空格和回车组成。 输入样例: Ji suan ke iiiii Suan Tou j un0 输出样例: 9 2 Jisuanke iiiii SuanToujun
可以使用两个变量分别记录空格和回车的数量,然后遍历字符串,遇到空格或回车就增加对应的变量。同时,可以使用另外一个字符串来存放处理后的结果,遇到回车就替换为空格,遇到空格就跳过。最后输出统计结果和处理后的字符串即可。
代码实现如下:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str[100];
cout << "请输入字符串:";
cin.getline(str, 100);
int spaceCount = 0, enterCount = 0;
char result[100];
int len = strlen(str);
int j = 0;
for (int i = 0; i < len; i++) {
if (str[i] == ' ') {
spaceCount++;
continue;
}
if (str[i] == '\r') {
enterCount++;
result[j++] = ' ';
continue;
}
result[j++] = str[i];
}
result[j] = '\0';
cout << "空格数量:" << spaceCount << endl;
cout << "回车数量:" << enterCount << endl;
cout << "处理后的字符串:" << result << endl;
return 0;
}
```
注意,这里使用了 `cin.getline()` 函数来读取含有空格和回车的字符串,而不是 `cin`。因为 `cin` 遇到空格或回车就会停止读取,而 `getline()` 可以读取整行字符串。
如何用C++编程来计算字符串中基于空格分隔的单词数目,当字符串表示为一个字符数组时?
在C++中,你可以通过遍历字符数组并检测空格来计算字符串中基于空格分隔的单词数目。下面是一个简单的示例:
```cpp
#include <iostream>
#include <cctype> // 包含处理字符类型的库
int countWords(char* str) {
int wordCount = 0; // 初始化词数为0
bool inWord = false; // 标记当前是否处于单词内部
for (char c : str) { // 遍历字符数组
if (c == ' ') { // 如果遇到空格
if (!inWord) continue; // 如果不在单词内部,则跳过
inWord = false;
wordCount++; // 增加词数
} else { // 非空格字符
if (isalpha(c)) { // 如果字符是字母
inWord = true; // 进入单词内部
}
}
}
// 别忘了检查最后一个单词(可能没有空格)
if (inWord) wordCount++;
return wordCount;
}
int main() {
char str[] = "Hello World, This is a test string.";
std::cout << "Number of words: " << countWords(str) << std::endl;
return 0;
}
```
在这个示例中,`countWords`函数首先初始化一个计数器`wordCount`,然后逐个检查字符。如果遇到空格并且之前不是在单词内部,就增加计数;如果是字母则认为还在单词中。最后,在遍历结束后,还要检查最后一个字符是否构成单词。
阅读全文
相关推荐
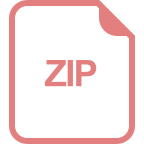
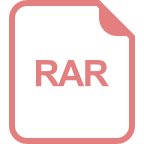
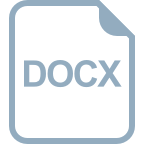
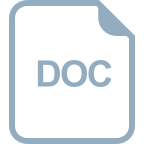









