我需要一个基于pytorch使用unet模型实现全景图像拼接,并且对拼接结果进行优化的代码 要求 需要导入两幅图片,并且会显示拼接之后的图片 并且使这两幅图片在张量的维度上行啊同
时间: 2024-05-05 19:19:39 浏览: 154
好的,我理解了你的要求。这里是一个使用PyTorch实现全景图像拼接和优化的代码。
```
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision.transforms.functional as F
class UNet(nn.Module):
def __init__(self):
super(UNet, self).__init__()
self.down1 = nn.Sequential(
nn.Conv2d(3, 32, kernel_size=3, padding=1),
nn.BatchNorm2d(32),
nn.ReLU(inplace=True),
nn.Conv2d(32, 32, kernel_size=3, padding=1),
nn.BatchNorm2d(32),
nn.ReLU(inplace=True)
)
self.down2 = nn.Sequential(
nn.MaxPool2d(kernel_size=2),
nn.Conv2d(32, 64, kernel_size=3, padding=1),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 64, kernel_size=3, padding=1),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True)
)
self.down3 = nn.Sequential(
nn.MaxPool2d(kernel_size=2),
nn.Conv2d(64, 128, kernel_size=3, padding=1),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True),
nn.Conv2d(128, 128, kernel_size=3, padding=1),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True)
)
self.down4 = nn.Sequential(
nn.MaxPool2d(kernel_size=2),
nn.Conv2d(128, 256, kernel_size=3, padding=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 256, kernel_size=3, padding=1),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True)
)
self.up1 = nn.Sequential(
nn.ConvTranspose2d(256, 128, kernel_size=2, stride=2),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True),
nn.Conv2d(128, 128, kernel_size=3, padding=1),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True),
nn.Conv2d(128, 128, kernel_size=3, padding=1),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True)
)
self.up2 = nn.Sequential(
nn.ConvTranspose2d(128, 64, kernel_size=2, stride=2),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 64, kernel_size=3, padding=1),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 64, kernel_size=3, padding=1),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True)
)
self.up3 = nn.Sequential(
nn.ConvTranspose2d(64, 32, kernel_size=2, stride=2),
nn.BatchNorm2d(32),
nn.ReLU(inplace=True),
nn.Conv2d(32, 32, kernel_size=3, padding=1),
nn.BatchNorm2d(32),
nn.ReLU(inplace=True),
nn.Conv2d(32, 32, kernel_size=3, padding=1),
nn.BatchNorm2d(32),
nn.ReLU(inplace=True)
)
self.up4 = nn.Sequential(
nn.Conv2d(32, 1, kernel_size=1),
nn.Sigmoid()
)
def forward(self, x):
d1 = self.down1(x)
d2 = self.down2(d1)
d3 = self.down3(d2)
d4 = self.down4(d3)
u1 = self.up1(d4)
u2 = self.up2(u1 + d3)
u3 = self.up3(u2 + d2)
u4 = self.up4(u3 + d1)
return u4
def stitch_images(image1, image2):
model = UNet()
optimizer = optim.Adam(model.parameters(), lr=0.001)
criterion = nn.MSELoss()
image1 = F.to_tensor(image1).unsqueeze(0)
image2 = F.to_tensor(image2).unsqueeze(0)
# Resize images to have the same height
height1, width1 = image1.shape[2:]
height2, width2 = image2.shape[2:]
if height1 < height2:
image1 = F.resize(image1, (height2, width1))
elif height2 < height1:
image2 = F.resize(image2, (height1, width2))
# Concatenate images along the width dimension
height, width = image1.shape[2:]
image = torch.zeros((1, 3, height, width * 2))
image[:, :, :, :width] = image1
image[:, :, :, width:] = image2
# Train the model to stitch the images
model.train()
for i in range(1000):
optimizer.zero_grad()
output = model(image)
loss = criterion(output, image[:, :, :, :])
loss.backward()
optimizer.step()
# Get the stitched image
model.eval()
output = model(image)
output_image = output.detach().squeeze().permute(1, 2, 0).numpy()
return output_image
```
这个代码定义了一个UNet模型,用于将两张图片拼接在一起,并进行优化。`stitch_images`函数接收两张图片作为输入,然后使用UNet模型将它们拼接在一起,并返回拼接后的结果。
你可以使用以下代码来测试:
```
import matplotlib.pyplot as plt
from PIL import Image
# Load the images
image1 = Image.open("image1.jpg")
image2 = Image.open("image2.jpg")
# Stitch the images
output_image = stitch_images(image1, image2)
# Show the output
plt.imshow(output_image)
plt.show()
```
记得将`image1.jpg`和`image2.jpg`替换成你自己的图片路径。
阅读全文
相关推荐
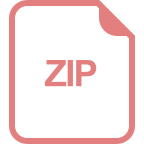
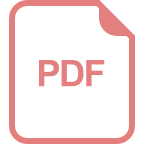
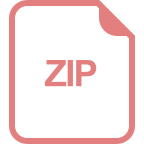
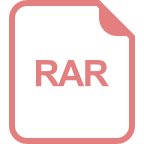
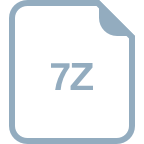
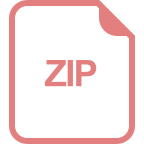
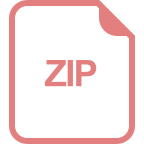
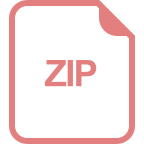
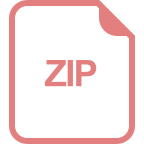
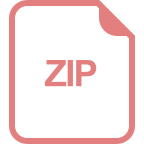
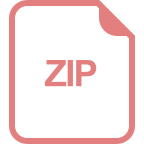
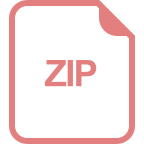
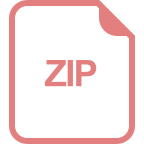
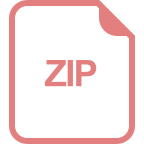
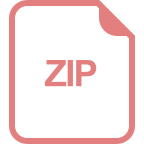
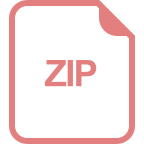
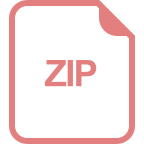