2.创建学生对象,包括姓名、年龄、住址、出生年月等属性;模拟生成学生集合,基于字段注解、打印输出该集合,要求输出格式为excel,且第一行数据为字段名称、后续行数据为集合数据; 2.1.定义注解类:用于标注集合对象属性;(10分) 2.2.定义工具类输出集合对象:集合输出时根据属性的标注输出属性字段名;(20分)
时间: 2023-06-08 18:04:30 浏览: 71
回答:好的,我明白了。您的第一个问题是关于如何创建和输出学生对象的集合的。根据您的要求,我将引入字段注解,并定义输出工具类来实现该功能。
首先,我们可以创建一个Student类,包含姓名、年龄、住址和出生年月等属性。然后,我们定义一个注解类,用于标注集合对象属性,例如:
```
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface FieldName {
String value();
}
```
该注解可以在字段上添加,用来表示该字段在输出时所对应的字段名称。
然后,可以实现一个工具类,用来将集合对象输出为Excel格式。该工具类可以接受一个集合对象作为参数,并依次遍历该集合对象的所有字段,将字段的值输出到Excel中。
在输出每个字段值之前,我们可以检查该字段上是否有@FieldName注解,若有,则取出注解的值作为该字段在输出时的名称。
示例代码如下:
```
public class ExcelExporter {
public static <T> void export(Collection<T> data, String[] headers, OutputStream os) {
Workbook wb = new HSSFWorkbook();
Sheet sheet = wb.createSheet("Sheet1");
Row row = sheet.createRow(0);
// 生成表头
for (int i = 0; i < headers.length; i++) {
Cell cell = row.createCell(i);
cell.setCellValue(headers[i]);
}
// 遍历集合输出数据
int rowIndex = 1;
for (T t : data) {
row = sheet.createRow(rowIndex++);
int i = 0;
for (Field f : t.getClass().getDeclaredFields()) {
f.setAccessible(true);
Object value = f.get(t);
String fieldName = f.getName();
if (f.isAnnotationPresent(FieldName.class)) {
fieldName = f.getAnnotation(FieldName.class).value();
}
Cell cell = row.createCell(i++);
if (value instanceof String) {
cell.setCellValue((String) value);
} else if (value instanceof Integer) {
cell.setCellValue((Integer) value);
} else if (value instanceof Long) {
cell.setCellValue((Long) value);
} else if (value instanceof Date) {
cell.setCellValue((Date) value);
}
}
}
wb.write(os);
}
}
```
以上就是一个简单的集合对象输出工具类,可以将集合对象输出为Excel格式。在输出时,会根据字段上的@FieldName注解生成表头名称。 示例如下:
```
public class Main {
public static void main(String[] args) throws FileNotFoundException {
List<Student> students = new ArrayList<>();
students.add(new Student("张三", 18, "北京市海淀区", new Date()));
students.add(new Student("李四", 19, "上海市浦东新区", new Date()));
students.add(new Student("王五", 20, "广州市天河区", new Date()));
String[] headers = new String[] { "姓名", "年龄", "住址", "出生年月" };
ExcelExporter.export(students, headers, new FileOutputStream("students.xls"));
}
}
class Student {
@FieldName("学生姓名")
String name;
@FieldName("学生年龄")
int age;
@FieldName("学生地址")
String address;
@FieldName("出生日期")
Date birthDate;
public Student(String name, int age, String address, Date birthDate) {
this.name = name;
this.age = age;
this.address = address;
this.birthDate = birthDate;
}
}
```
运行该代码后,会在当前目录下生成一个名为students.xls的Excel文件,文件中包含了学生对象的集合,并且字段名与上面@FieldName注解中定义的相同: 学生姓名, 学生年龄, 学生地址, 出生日期。
相关推荐
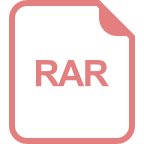
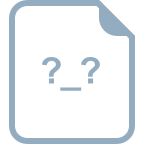
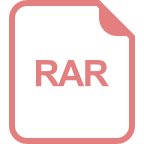














