RuntimeError: Input type (torch.cuda.DoubleTensor) and weight type (torch.cuda.FloatTensor) should be the same
时间: 2023-11-05 09:29:19 浏览: 191
这个错误提示是因为输入张量的数据类型和模型参数的数据类型不一致,需要将它们转换为相同的数据类型。可以使用`.to()`方法将输入张量或模型参数转换为相同的数据类型,示例代码如下:
```python
import torch
# 定义设备
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
# 定义模型
model = nn.Linear(10, 1)
# 将模型移动到GPU上
model.to(device)
# 定义输入张量
input_tensor = torch.randn(2, 10).double()
# 将输入张量移动到GPU上并转换为float类型
input_tensor = input_tensor.to(device, dtype=torch.float)
# 进行模型计算
output = model(input_tensor)
# 输出结果
print(output)
```
在上面的代码中,我们将输入张量`input_tensor`转换为float类型,并将其移动到GPU上。这样,输入张量的数据类型和模型参数的数据类型就一致了,就可以避免该错误的出现。
相关问题
RuntimeError: Input type (torch.cuda.DoubleTensor) and weight type (torch.DoubleTensor) should be the same
这个错误提示表明输入类型(torch.cuda.DoubleTensor)和权重类型(torch.DoubleTensor)不一致,需要将它们转换为相同的类型。可以使用.to()方法将输入和权重都转换为相同的类型,例如:
```
input = input.to(torch.double)
weight = weight.to(torch.double)
```
如果你使用的是GPU,还需要将它们都移动到相同的设备上,例如:
```
input = input.to(device)
weight = weight.to(device)
```
其中,device是你当前使用的设备,可以使用torch.device()方法指定。
RuntimeError: Input type (torch.cuda.FloatTensor) and weight type (torch.FloatTensor) should be the same
这个错误通常是由于输入的数据类型与模型权重的数据类型不一致导致的。可能是你的输入数据是在GPU上运行的(torch.cuda.FloatTensor),但是模型权重是在CPU上(torch.FloatTensor)。你可以尝试将输入数据转换为与模型权重相同的数据类型,比如使用`input_tensor.to('cpu')`或`input_tensor.to('cuda')`将输入数据放到相应的设备上。你还可以尝试在模型的初始化中指定权重类型,比如`model = MyModel().to(torch.device('cuda')).float()`。
阅读全文
相关推荐
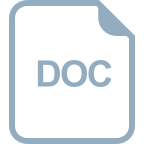
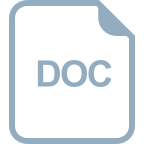
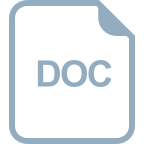

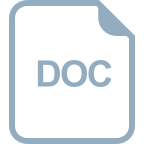
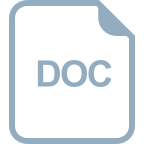
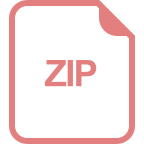
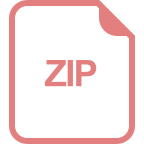
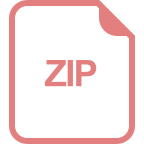
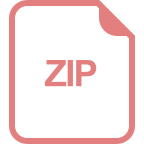
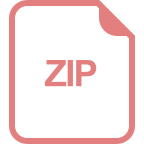