sws_scale(sws_ctx, pframe->data, pframe->linesize, // 0, pcodec_ctx->height, pframe_rgb->data, pframe_rgb->linesize);
时间: 2024-04-01 11:36:18 浏览: 133
这是一段视频处理的代码,使用了FFmpeg库中的sws_scale函数,将pframe中的视频帧数据转换为RGB格式,并存储到pframe_rgb中。其中,sws_ctx是由sws_getContext函数生成的一个用于视频转换的上下文结构体,pframe是原始的视频帧数据,pframe_rgb是转换后的RGB格式视频帧数据。pframe->data和pframe->linesize分别指向原始视频帧数据的存储地址和每行数据的大小,0和pcodec_ctx->height分别表示转换后视频帧数据的起始行和高度。pframe_rgb->data和pframe_rgb->linesize分别指向转换后RGB格式视频帧数据的存储地址和每行数据的大小。
相关问题
生成一个qt下使用opencv 进行 rtmp推流 其中包含 rtmp_sendpacket 例程
很高兴能为您提供帮助,以下是生成一个Qt下使用OpenCV进行rtmp推流的步骤和例程:
步骤:
1.安装OpenCV,可以从OpenCV官网下载对应的版本进行安装。
2.下载并安装FFmpeg,可以从FFmpeg官网下载对应的版本进行安装。
3.在Qt中配置OpenCV和FFmpeg,在项目.pro文件中添加以下代码:
```
INCLUDEPATH += [OpenCV的include目录]
LIBS += -L[OpenCV的lib目录] -lopencv_core -lopencv_highgui -lopencv_imgproc -lopencv_videoio -lopencv_imgcodecs
INCLUDEPATH += [FFmpeg的include目录]
LIBS += -L[FFmpeg的lib目录] -lavcodec -lavdevice -lavfilter -lavformat -lavutil -lswresample -lswscale -lavcodec
```
4.编写rtmp推流代码,示例代码如下:
```
#include <opencv2/opencv.hpp>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/opt.h>
#include <libavutil/imgutils.h>
#include <libswscale/swscale.h>
#define STREAM_URL "rtmp://[推流地址]"
using namespace cv;
int main()
{
AVFormatContext *pFormatCtx;
AVOutputFormat *fmt;
AVStream *video_st;
AVCodecContext *pCodecCtx;
AVCodec *pCodec;
AVFrame *pFrame, *pFrameRGB;
uint8_t *buffer;
int videoindex = -1;
int framecnt = 0;
int64_t start_time = 0;
struct SwsContext *img_convert_ctx;
// OpenCV读取视频文件
VideoCapture capture(0);
if(!capture.isOpened())
{
printf("OpenCV: Could not open camera.\n");
return -1;
}
// 初始化FFmpeg
av_register_all();
// 初始化输出格式
avformat_alloc_output_context2(&pFormatCtx, NULL, "flv", STREAM_URL);
if(!pFormatCtx)
{
printf("FFmpeg: Could not allocate output context.\n");
return -1;
}
fmt = pFormatCtx->oformat;
// 添加视频流
video_st = avformat_new_stream(pFormatCtx, 0);
if(!video_st)
{
printf("FFmpeg: Could not create new stream.\n");
return -1;
}
videoindex = video_st->index;
// 设置编码器参数
pCodecCtx = video_st->codec;
pCodecCtx->codec_id = fmt->video_codec;
pCodecCtx->codec_type = AVMEDIA_TYPE_VIDEO;
pCodecCtx->pix_fmt = AV_PIX_FMT_YUV420P;
pCodecCtx->width = capture.get(CV_CAP_PROP_FRAME_WIDTH);
pCodecCtx->height = capture.get(CV_CAP_PROP_FRAME_HEIGHT);
pCodecCtx->time_base.num = 1;
pCodecCtx->time_base.den = 25;
// 查找编码器
pCodec = avcodec_find_encoder(pCodecCtx->codec_id);
if(!pCodec)
{
printf("FFmpeg: Could not find encoder.\n");
return -1;
}
// 打开编码器
if(avcodec_open2(pCodecCtx, pCodec, NULL) < 0)
{
printf("FFmpeg: Could not open encoder.\n");
return -1;
}
// 分配视频帧内存
pFrame = av_frame_alloc();
pFrameRGB = av_frame_alloc();
// 分配视频帧缓冲区内存
int numBytes = av_image_get_buffer_size(AV_PIX_FMT_YUV420P, pCodecCtx->width, pCodecCtx->height, 1);
buffer = (uint8_t *)av_malloc(numBytes * sizeof(uint8_t));
av_image_fill_arrays(pFrame->data, pFrame->linesize, buffer, AV_PIX_FMT_YUV420P, pCodecCtx->width, pCodecCtx->height, 1);
// 分配视频帧RGB缓冲区内存
uint8_t *rgbBuffer = (uint8_t *)av_malloc(av_image_get_buffer_size(AV_PIX_FMT_RGB24, pCodecCtx->width, pCodecCtx->height, 1));
av_image_fill_arrays(pFrameRGB->data, pFrameRGB->linesize, rgbBuffer, AV_PIX_FMT_RGB24, pCodecCtx->width, pCodecCtx->height, 1);
// 初始化图像转换上下文
img_convert_ctx = sws_getContext(pCodecCtx->width, pCodecCtx->height, AV_PIX_FMT_RGB24, pCodecCtx->width, pCodecCtx->height, AV_PIX_FMT_YUV420P, SWS_BICUBIC, NULL, NULL, NULL);
// 输出格式信息
av_dump_format(pFormatCtx, 0, STREAM_URL, 1);
// 打开输出URL
if(!(fmt->flags & AVFMT_NOFILE))
{
if(avio_open(&pFormatCtx->pb, STREAM_URL, AVIO_FLAG_WRITE) < 0)
{
printf("FFmpeg: Could not open output URL.\n");
return -1;
}
}
// 写入头部
avformat_write_header(pFormatCtx, NULL);
while(capture.read(pFrameRGB->data[0]))
{
// RGB转YUV
sws_scale(img_convert_ctx, pFrameRGB->data, pFrameRGB->linesize, 0, pCodecCtx->height, pFrame->data, pFrame->linesize);
// 视频帧时间
AVRational time_base = {1, 1000};
int64_t pts = framecnt * (pCodecCtx->time_base.den * 1000 / pCodecCtx->time_base.num) / 25;
pFrame->pts = av_rescale_q(pts, time_base, video_st->time_base);
pFrame->key_frame = 1;
// 编码并推送视频帧
AVPacket pkt;
int ret = avcodec_send_frame(pCodecCtx, pFrame);
if(ret < 0)
{
printf("FFmpeg: Error sending frame.\n");
break;
}
while(ret >= 0)
{
ret = avcodec_receive_packet(pCodecCtx, &pkt);
if(ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
{
break;
}
else if(ret < 0)
{
printf("FFmpeg: Error encoding frame.\n");
return -1;
}
// 发送视频帧Packet
av_packet_rescale_ts(&pkt, pCodecCtx->time_base, video_st->time_base);
pkt.stream_index = videoindex;
ret = av_interleaved_write_frame(pFormatCtx, &pkt);
av_packet_unref(&pkt);
}
// 输出进度信息
printf("FFmpeg: Encode frame index:%d\n", framecnt);
framecnt++;
// 推流时间
if(start_time == 0)
{
start_time = av_gettime();
}
int64_t now_time = av_gettime() - start_time;
int64_t duration = framecnt * pCodecCtx->time_base.num * 1000 / pCodecCtx->time_base.den;
if(now_time < duration)
{
av_usleep(duration - now_time);
}
}
// 写入尾部
av_write_trailer(pFormatCtx);
// 释放内存
avcodec_close(pCodecCtx);
av_free(pCodecCtx);
av_frame_free(&pFrame);
av_frame_free(&pFrameRGB);
av_free(buffer);
av_free(rgbBuffer);
avio_close(pFormatCtx->pb);
avformat_free_context(pFormatCtx);
return 0;
}
```
注:在实际使用中需要将[推流地址]替换为实际的地址。
这是一个简单的例程,仅供参考,具体实现方式还需要根据实际情况进行调整和完善。
阅读全文
相关推荐
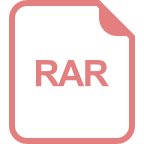
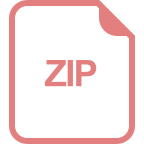
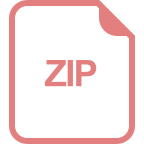
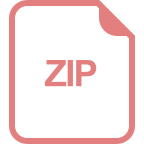
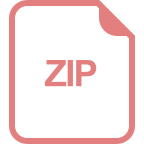
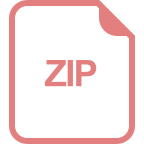
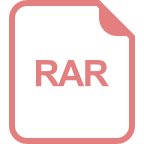
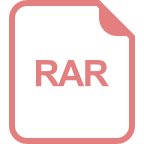
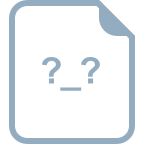
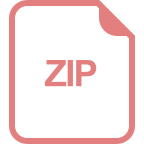
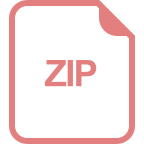
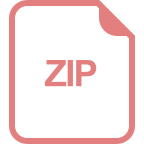
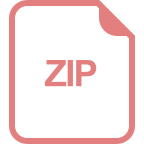