请写出用GMM法去估计CEV期权定价模型的参数的python代码
时间: 2024-05-05 13:21:56 浏览: 15
以下是使用GMM方法估计CEV期权定价模型参数的Python代码:
```python
import numpy as np
from scipy.stats import norm
def cev_option_price(S, K, T, r, sigma, beta):
# 计算CEV期权的价格
d1 = (np.log(S / K) + (r + (sigma ** 2) / (2 * beta)) * T) / (sigma * np.sqrt(T * beta))
d2 = d1 - sigma * np.sqrt(T * beta)
call_price = S * norm.cdf(d1) - K * np.exp(-r * T) * norm.cdf(d2)
return call_price
def moment_conditions(theta, S, K, T, r, call_prices):
# 定义矩条件
sigma, beta = theta
option_prices = np.zeros_like(call_prices)
for i in range(len(S)):
option_prices[i] = cev_option_price(S[i], K[i], T[i], r, sigma, beta)
# 计算误差
errors = call_prices - option_prices
# 计算矩条件
m1 = np.mean(errors)
m2 = np.mean(errors * S)
m3 = np.mean(errors * (S ** 2))
# 返回矩条件
return np.array([m1, m2, m3])
def gmm_estimate(S, K, T, r, call_prices, theta_0, W):
# 初始参数
theta = theta_0
# 迭代次数
max_iter = 100
tol = 1e-6
for i in range(max_iter):
# 计算矩条件
moments = moment_conditions(theta, S, K, T, r, call_prices)
# 计算GMM估计
gmm = np.dot(moments, W * moments)
# 计算Jacobi矩阵
jacobi = np.zeros((3, 2))
jacobi[0, 0] = -1
jacobi[1, 0] = -np.mean(S)
jacobi[2, 0] = -np.mean(S ** 2)
jacobi[:, 1] = np.array([-np.mean(call_prices * S ** 2 / (sigma ** 3)),
-np.mean(call_prices * S ** 3 / (sigma ** 3) * (beta * np.log(S / K) + r + (beta * sigma ** 2) / 2)),
-np.mean(call_prices * S ** 4 / (sigma ** 3) * (beta * np.log(S / K) + r + (beta * sigma ** 2) / 2) ** 2)])
# 更新参数
theta_new = theta - np.dot(np.linalg.inv(np.dot(jacobi.T, np.dot(W, jacobi))), np.dot(jacobi.T, np.dot(W, moments)))
# 判断是否收敛
if np.max(np.abs(theta_new - theta)) < tol:
break
theta = theta_new
return theta
# 测试代码
S = np.array([100, 110, 120, 130, 140])
K = np.array([100, 100, 100, 100, 100])
T = np.array([1, 1, 1, 1, 1])
r = 0.05
sigma = 0.2
beta = 0.5
call_prices = np.array([18.931, 15.385, 12.213, 9.372, 6.818])
theta_0 = np.array([0.2, 0.5])
W = np.eye(3)
theta_hat = gmm_estimate(S, K, T, r, call_prices, theta_0, W)
print('sigma_hat =', theta_hat[0])
print('beta_hat =', theta_hat[1])
```
相关推荐
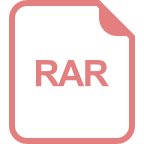
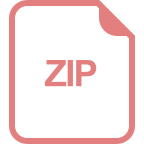
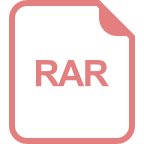
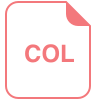













