用C语言写一个串口收发数据的demo
时间: 2024-05-08 18:19:09 浏览: 94
好的,以下是一个简单的C语言串口收发数据的demo:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define BAUDRATE B9600
#define MODEMDEVICE "/dev/ttyS0"
int set_serial()
{
int fd;
struct termios oldtio,newtio;
fd = open(MODEMDEVICE, O_RDWR | O_NOCTTY);
if (fd < 0) {
perror(MODEMDEVICE);
exit(1);
}
tcgetattr(fd, &oldtio);
memset(&newtio, 0, sizeof(newtio));
newtio.c_cflag = BAUDRATE | CS8 | CLOCAL | CREAD;
newtio.c_iflag = IGNPAR;
newtio.c_oflag = 0;
newtio.c_lflag = 0;
newtio.c_cc[VTIME] = 0;
newtio.c_cc[VMIN] = 1;
tcflush(fd, TCIFLUSH);
tcsetattr(fd, TCSANOW, &newtio);
return fd;
}
int main()
{
int fd = set_serial();
char buf[255];
while (1) {
memset(buf, 0, sizeof(buf));
read(fd, buf, 255);
printf("Received: %s\n", buf);
usleep(1000);
}
close(fd);
return 0;
}
```
该demo打开了串口设备/dev/ttyS0,并设置了波特率为9600,数据位为8位,无校验位,停止位为1位。通过while循环不断读取串口接收到的数据,并打印到终端上。
希望这个例子对你有所帮助。
阅读全文
相关推荐
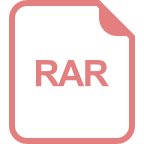
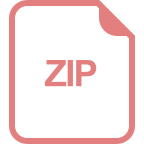
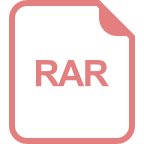
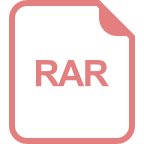
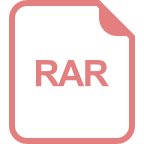
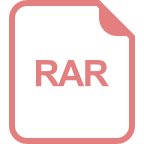
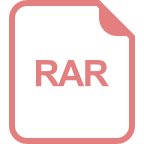
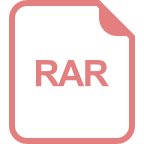
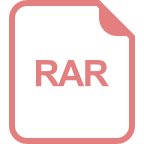
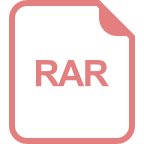
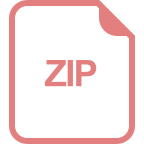
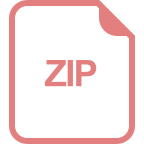
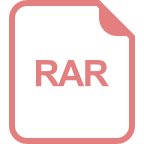
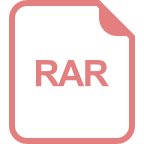
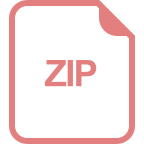
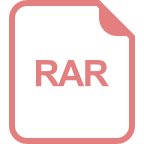
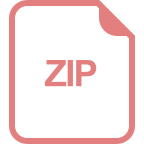