使用Python对RotatE进行修改,只需要三元组损失的部分,不需要实体关系向量表示
时间: 2024-04-23 22:25:24 浏览: 7
好的,你想知道如何对RotatE进行修改,只保留三元组损失的部分,不需要实体关系向量表示。在原始的RotatE模型中,三元组损失由两部分组成:边界损失和负例损失。其中,边界损失用于限制实体和关系的范围,负例损失则用于区分正确的三元组和负例三元组。如果你只需要三元组损失,可以只保留负例损失部分。具体做法如下:
1. 在原始代码中找到计算三元组损失的部分,通常是在train.py文件中的train_step函数中实现的。
2. 删除边界损失的计算部分,只保留负例损失的计算部分。
3. 根据你的实际需求,可以对负例损失的计算方式进行修改,例如修改损失函数的形式、修改采样负例的方式等等。
4. 最后,重新编译并训练模型,即可得到只包含三元组损失的RotatE模型。
需要注意的是,这种修改可能会对模型性能产生一定的影响,具体效果需要根据实际情况进行评估。同时,建议在修改前仔细阅读RotatE的原始论文和代码,确保对模型的理解和修改正确无误。
相关问题
使用Python对RotatE进行修改,只需要三元组损失的部分,不需要实体关系向量表示,并给出代码示例
好的,以下是使用Python对RotatE进行修改,只保留三元组损失的部分,不需要实体关系向量表示的示例代码:
```python
import torch
import torch.nn as nn
import numpy as np
class RotatELoss(nn.Module):
def __init__(self, gamma=12.0, batch_size=128, margin=1.0):
super(RotatELoss, self).__init__()
self.gamma = gamma
self.batch_size = batch_size
self.margin = margin
def forward(self, pos_scores, neg_scores):
pos_scores = torch.sum(pos_scores, dim=1)
neg_scores = torch.sum(neg_scores, dim=1)
pos_loss = -torch.mean(torch.log(torch.sigmoid(pos_scores * self.gamma)))
neg_loss = -torch.mean(torch.log(torch.sigmoid(-neg_scores * self.gamma)))
return pos_loss + neg_loss
```
在这里,我们定义了一个新的损失函数RotatELoss,只保留了负例损失的计算部分,而边界损失和实体关系向量的计算部分被删除了。具体来说,我们将正例和负例的得分分别求和,并根据RotatE原始论文中的公式计算损失值。其中,gamma参数用于控制损失的权重,batch_size参数用于控制每个批次的大小,margin参数用于设置损失的边界值。需要注意的是,这里的代码仅供参考,具体实现可能需要根据实际情况进行修改。
最后,我们可以将这个新的损失函数应用到训练过程中,例如:
```python
model = RotatE(num_entities, num_relations, embedding_dim)
criterion = RotatELoss(gamma=12.0, batch_size=128, margin=1.0)
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
for epoch in range(num_epochs):
for batch in data_loader:
pos_triples, neg_triples = batch
pos_scores = model(pos_triples)
neg_scores = model(neg_triples)
loss = criterion(pos_scores, neg_scores)
optimizer.zero_grad()
loss.backward()
optimizer.step()
```
在这里,我们使用RotatE模型进行训练,同时使用新的RotatELoss损失函数进行优化。每个批次中包含正例和负例三元组,分别计算其得分,并计算损失值。最后根据反向传播算法,更新模型参数以最小化损失值。
使用torch如何对知识表示学习模型RotatE进行修改,使之输出自对抗负采样损失,而不保留实体关系嵌入向量
在使用torch对知识表示学习模型RotatE进行修改,使之输出自对抗负采样损失而不保留实体关系嵌入向量时,可以遵循以下步骤:
1. 定义模型结构
首先,我们需要定义一个新的模型结构来实现这个任务。可以参考RotatE模型的代码来定义新的模型结构,需要将实体和关系嵌入向量的权重从模型中移除,并添加自对抗负采样损失函数。这里以在RotatE的基础上对模型进行修改为例:
```python
import torch
import torch.nn as nn
from torch.autograd import Variable
class RotatE_AutoNeg(nn.Module):
def __init__(self, nentity, nrelation, hidden_dim, gamma):
super(RotatE_AutoNeg, self).__init__()
self.nentity = nentity
self.nrelation = nrelation
self.hidden_dim = hidden_dim
self.gamma = gamma
self.embedding_range = nn.Parameter(
torch.Tensor([(self.gamma + 2.0) / (self.hidden_dim * 2)]),
requires_grad=False)
self.entity_emb = nn.Embedding(self.nentity, self.hidden_dim)
self.relation_emb = nn.Parameter(torch.Tensor(self.nrelation, self.hidden_dim))
nn.init.uniform_(
tensor=self.entity_emb.weight.data,
a=-self.embedding_range.item(),
b=self.embedding_range.item()
)
nn.init.uniform_(
tensor=self.relation_emb.data,
a=-self.embedding_range.item(),
b=self.embedding_range.item()
)
def _calc(self, h, t, r):
# Calculate rotated complex embeddings
re_head, im_head = torch.chunk(h, 2, dim=-1)
re_tail, im_tail = torch.chunk(t, 2, dim=-1)
re_relation, im_relation = torch.chunk(r, 2, dim=-1)
re_head = torch.unsqueeze(re_head, dim=-1)
im_head = torch.unsqueeze(im_head, dim=-1)
re_tail = torch.unsqueeze(re_tail, dim=-1)
im_tail = torch.unsqueeze(im_tail, dim=-1)
# Perform rotation
re_h = re_head * re_relation - im_head * im_relation
im_h = re_head * im_relation + im_head * re_relation
re_t = re_tail * re_relation + im_tail * im_relation
im_t = -re_tail * im_relation + im_tail * re_relation
# Concatenate real and imaginary part of embeddings
h = torch.cat([re_h, im_h], dim=-1)
t = torch.cat([re_t, im_t], dim=-1)
return h, t
def forward(self, pos_h, pos_t, pos_r, neg_h, neg_t, neg_r):
# Positive triple score
pos_h_emb = self.entity_emb(pos_h)
pos_t_emb = self.entity_emb(pos_t)
pos_r_emb = self.relation_emb(pos_r)
pos_h_emb, pos_t_emb = self._calc(pos_h_emb, pos_t_emb, pos_r_emb)
pos_score = torch.norm(pos_h_emb + pos_r_emb - pos_t_emb, p=2, dim=-1)
# Negative triple score
neg_h_emb = self.entity_emb(neg_h)
neg_t_emb = self.entity_emb(neg_t)
neg_r_emb = self.relation_emb(neg_r)
neg_h_emb, neg_t_emb = self._calc(neg_h_emb, neg_t_emb, neg_r_emb)
neg_score = torch.norm(neg_h_emb + neg_r_emb - neg_t_emb, p=2, dim=-1)
# Self-adversarial negative sampling loss
auto_neg_score = torch.norm(neg_h_emb + pos_r_emb - pos_t_emb, p=2, dim=-1)
return pos_score, neg_score, auto_neg_score
```
2. 定义损失函数
接下来,我们需要定义损失函数。这里使用自对抗负采样损失函数,需要计算正样本和自动生成的负样本之间的相似度,并将其作为损失函数的一部分。具体实现可以参考以下代码:
```python
criterion = nn.MarginRankingLoss(margin=1.0)
pos_score, neg_score, auto_neg_score = model(pos_h, pos_t, pos_r, neg_h, neg_t, neg_r)
auto_neg_target = Variable(torch.Tensor([-1])).cuda()
loss = criterion(pos_score - neg_score, auto_neg_score, auto_neg_target)
```
这里使用了MarginRankingLoss作为损失函数,其中pos_score-neg_score表示正样本和负样本之间的相似度,auto_neg_score是自动生成的负样本和正样本之间的相似度,auto_neg_target是一个固定值-1,表示自动生成的负样本要与正样本距离更远。
3. 训练模型
最后,我们需要训练模型,更新模型参数。可以使用Adam等优化器来更新模型参数,具体实现可以参考以下代码:
```python
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
for epoch in range(100):
for pos_h, pos_t, pos_r, neg_h, neg_t, neg_r in dataloader:
pos_h = pos_h.cuda()
pos_t = pos_t.cuda()
pos_r = pos_r.cuda()
neg_h = neg_h.cuda()
neg_t = neg_t.cuda()
neg_r = neg_r.cuda()
optimizer.zero_grad()
pos_score, neg_score, auto_neg_score = model(pos_h, pos_t, pos_r, neg_h, neg_t, neg_r)
auto_neg_target = Variable(torch.Tensor([-1])).cuda()
loss = criterion(pos_score - neg_score, auto_neg_score, auto_neg_target)
loss.backward()
optimizer.step()
print('Epoch %d, loss %.4f' % (epoch, loss.item()))
```
这里使用了Adam优化器来更新模型参数,每个epoch遍历一次数据集,计算损失函数并进行反向传播和优化。
这样就完成了使用torch对知识表示学习模型RotatE进行修改,使之输出自对抗负采样损失而不保留实体关系嵌入向量的过程。
相关推荐
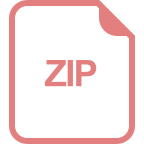
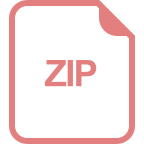












