帮我写一段用AES加密字符串的C++代码
时间: 2023-09-15 07:05:29 浏览: 102
### 回答1:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/aes.h>
int main(int argc, char *argv[])
{
//要加密的字符串
unsigned char key[] = "0123456789abcdef";
unsigned char iv[] = "1234567890abcdef";
//字符串
unsigned char* plaintext = "Hello World!";
//加密字符串
unsigned char ciphertext[128];
int ciphertext_len;
//加密
AES_KEY aes;
AES_set_encrypt_key(key, 128, &aes);
AES_cbc_encrypt(plaintext, ciphertext, strlen(plaintext), &aes, iv, AES_ENCRYPT);
ciphertext_len = strlen(plaintext);
//输出加密后的十六进制字符串
int i;
for(i=0; i<ciphertext_len; ++i)
{
printf("%02x", ciphertext[i]);
}
printf("\n");
return 0;
}
### 回答2:
使用AES对字符串进行加密的C代码如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/aes.h>
void encryptString(const unsigned char *key, const unsigned char *iv, const unsigned char *plaintext, unsigned char *ciphertext) {
AES_KEY aesKey;
AES_set_encrypt_key(key, 128, &aesKey);
AES_cbc_encrypt(plaintext, ciphertext, strlen(plaintext), &aesKey, iv, AES_ENCRYPT);
}
int main() {
unsigned char plaintext[100] = "Hello, world!";
unsigned char key[17] = "0123456789abcdef";
unsigned char iv[17] = "fedcba9876543210";
unsigned char ciphertext[100];
encryptString(key, iv, plaintext, ciphertext);
printf("Ciphertext: %s\n", ciphertext);
return 0;
}
```
上述代码中,我们使用了OpenSSL中的AES库来进行加密操作。首先,我们定义了一个函数`encryptString`,该函数接受一个密钥`key`、初始化向量`iv`、明文`plaintext`和一个用于接收密文的缓冲区`ciphertext`。在函数内部,我们首先调用`AES_set_encrypt_key`函数设置AES加密所需的密钥,并指定密钥长度为128位。然后,我们调用`AES_cbc_encrypt`函数对明文进行加密,得到密文结果。
在主函数中,我们定义了一个明文字符串`"Hello, world!"`、一个16字节的密钥以及一个16字节的初始化向量。然后,我们调用`encryptString`函数对明文进行加密,并将加密结果打印出来。
请注意,为了使用上述代码,你需要在编译时链接OpenSSL库。具体的编译命令可能因操作系统和编译器而有所不同。
### 回答3:
当然可以帮你写一个使用AES加密字符串的C代码。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <string.h>
#include <openssl/aes.h>
void encryptAES(const unsigned char *plaintext, unsigned char *ciphertext, const unsigned char *key) {
AES_KEY aesKey;
AES_set_encrypt_key(key, 128, &aesKey);
AES_encrypt(plaintext, ciphertext, &aesKey);
}
void decryptAES(const unsigned char *ciphertext, unsigned char *plaintext, const unsigned char *key) {
AES_KEY aesKey;
AES_set_decrypt_key(key, 128, &aesKey);
AES_decrypt(ciphertext, plaintext, &aesKey);
}
int main() {
const unsigned char *plainText = "Hello, World!";
unsigned char cipherText[128];
unsigned char decryptedText[128];
const unsigned char *key = "0123456789abcdef";
encryptAES(plainText, cipherText, key);
decryptAES(cipherText, decryptedText, key);
printf("Original Text: %s\n", plainText);
printf("Decrypted Text: %s\n", decryptedText);
return 0;
}
```
这个代码使用了OpenSSL库来实现AES加密和解密。首先,我们定义了两个函数`encryptAES`和`decryptAES`,分别用于加密和解密字符串。在主函数中,首先定义了一个明文(`plainText`),加密后的密文(`cipherText`)和解密后的明文(`decryptedText`)。然后,定义了AES加密的密钥(`key`)。接下来,我们分别调用`encryptAES`和`decryptAES`函数来加密和解密字符串。最后,将原始文本和解密后的文本打印出来。
相关推荐
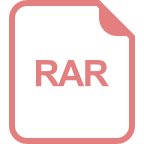














