C语言什么时候应该加getchar()吃掉回车
时间: 2023-06-13 10:04:23 浏览: 63
在C语言中,当使用scanf()函数读入字符或字符串时,输入缓冲区中的回车符('\n')也会被读入。这可能导致后续的输入函数跳过输入或出现不必要的输入,因此需要使用getchar()函数吃掉缓冲区中的回车符。具体来说,在读取字符或字符串后,应该使用getchar()函数吃掉输入缓冲区中的回车符,如下所示:
```
char c;
scanf("%c", &c);
getchar(); //吃掉回车符
```
```
char str[100];
scanf("%s", str);
getchar(); //吃掉回车符
```
需要注意的是,如果在读取数字时使用scanf()函数,回车符会被自动忽略,无需使用getchar()函数吃掉。
相关问题
C语言编写代码:题目描述 给你一个随机生成的字符串,其中不包括换行,你的任务是倒着把它输出出来 输入 第一行一个正整数n,表示字符串的长度,不超过100 第二行开始,一个字符串(字符数组) 输出 倒着输出它想想字符包括了什么? 参考代码模板: #include <stdio.h> int main() { int n; scanf("%d",&n); getchar();//吃掉回车 char s[100]; gets(s); //补充代码倒着输出s
好的,以下是基于 C 语言的代码实现:
```c
#include <stdio.h>
#include <string.h>
int main() {
int n;
scanf("%d", &n); // 读入字符串的长度
getchar(); // 吃掉回车
char s[101];
gets(s); // 读入字符串
int len = strlen(s);
for(int i = len - 1; i >= 0; i--) {
printf("%c", s[i]); // 倒着输出字符串
}
return 0;
}
```
代码解析:
首先读入字符串的长度 `n`,然后使用 `getchar` 函数吃掉回车符。接着定义一个字符数组 `s`,用于存储读入的字符串。然后使用 `gets` 函数读入字符串,`gets` 函数可以读入包含空格在内的整行字符串。读入的字符串将会存储在字符数组 `s` 中。
接下来,使用 `strlen` 函数获取字符串的长度 `len`,然后使用 `for` 循环倒序输出字符串。注意,字符数组的下标是从 0 开始的,因此倒序输出时循环变量 `i` 的初始值是 `len - 1`,循环结束的条件是 `i >= 0`,每次循环时输出字符数组 `s` 中下标为 `i` 的字符即可。
需要注意的是,由于输入的字符串不包含换行符,因此使用 `gets` 函数读入字符串时不需要使用 `fgets` 函数。另外,由于题目中规定字符串的长度不超过 100,因此定义字符数组 `s` 时需要分配 101 个元素,以便存储字符串的每个字符以及字符串结束标志符 `\0`。
codeblocks的C语言围棋双人对战程序包含吃子,计算外气,禁手规则以及自动提子的代码
以下是一个简单的围棋双人对战程序,包含吃子、计算外气、禁手规则以及自动提子的代码。这个程序使用Code::Blocks和C语言编写。
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define BOARD_SIZE 9
#define EMPTY 0
#define BLACK 1
#define WHITE 2
int board[BOARD_SIZE][BOARD_SIZE];
int current_player = BLACK;
bool is_legal_move(int x, int y) {
if (board[x][y] != EMPTY) {
return false;
}
// 判断是否为自杀
board[x][y] = current_player;
bool has_liberty = false;
if (x > 0 && board[x-1][y] != EMPTY) {
has_liberty = true;
}
if (y > 0 && board[x][y-1] != EMPTY) {
has_liberty = true;
}
if (x < BOARD_SIZE-1 && board[x+1][y] != EMPTY) {
has_liberty = true;
}
if (y < BOARD_SIZE-1 && board[x][y+1] != EMPTY) {
has_liberty = true;
}
if (!has_liberty) {
board[x][y] = EMPTY;
return false;
}
// 判断是否为禁手
if (x > 1 && board[x-1][y] == current_player && board[x-2][y] == current_player) {
board[x][y] = EMPTY;
return false;
}
if (y > 1 && board[x][y-1] == current_player && board[x][y-2] == current_player) {
board[x][y] = EMPTY;
return false;
}
if (x < BOARD_SIZE-2 && board[x+1][y] == current_player && board[x+2][y] == current_player) {
board[x][y] = EMPTY;
return false;
}
if (y < BOARD_SIZE-2 && board[x][y+1] == current_player && board[x][y+2] == current_player) {
board[x][y] = EMPTY;
return false;
}
// 判断是否吃子
if (x > 0 && board[x-1][y] == 3-current_player) {
bool has_liberty = false;
if (x > 1 && board[x-2][y] != EMPTY) {
has_liberty = true;
}
if (y > 0 && board[x-1][y-1] != EMPTY) {
has_liberty = true;
}
if (y < BOARD_SIZE-1 && board[x-1][y+1] != EMPTY) {
has_liberty = true;
}
if (!has_liberty) {
board[x][y] = EMPTY;
board[x-1][y] = EMPTY;
return true;
}
}
if (y > 0 && board[x][y-1] == 3-current_player) {
bool has_liberty = false;
if (x > 0 && board[x-1][y-1] != EMPTY) {
has_liberty = true;
}
if (x < BOARD_SIZE-1 && board[x+1][y-1] != EMPTY) {
has_liberty = true;
}
if (y > 1 && board[x][y-2] != EMPTY) {
has_liberty = true;
}
if (!has_liberty) {
board[x][y] = EMPTY;
board[x][y-1] = EMPTY;
return true;
}
}
if (x < BOARD_SIZE-1 && board[x+1][y] == 3-current_player) {
bool has_liberty = false;
if (x < BOARD_SIZE-2 && board[x+2][y] != EMPTY) {
has_liberty = true;
}
if (y > 0 && board[x+1][y-1] != EMPTY) {
has_liberty = true;
}
if (y < BOARD_SIZE-1 && board[x+1][y+1] != EMPTY) {
has_liberty = true;
}
if (!has_liberty) {
board[x][y] = EMPTY;
board[x+1][y] = EMPTY;
return true;
}
}
if (y < BOARD_SIZE-1 && board[x][y+1] == 3-current_player) {
bool has_liberty = false;
if (x > 0 && board[x-1][y+1] != EMPTY) {
has_liberty = true;
}
if (x < BOARD_SIZE-1 && board[x+1][y+1] != EMPTY) {
has_liberty = true;
}
if (y < BOARD_SIZE-2 && board[x][y+2] != EMPTY) {
has_liberty = true;
}
if (!has_liberty) {
board[x][y] = EMPTY;
board[x][y+1] = EMPTY;
return true;
}
}
board[x][y] = EMPTY;
return true;
}
int count_liberties(int x, int y) {
if (board[x][y] == EMPTY) {
return 0;
}
int liberties = 0;
if (x > 0 && board[x-1][y] == EMPTY) {
liberties++;
}
if (y > 0 && board[x][y-1] == EMPTY) {
liberties++;
}
if (x < BOARD_SIZE-1 && board[x+1][y] == EMPTY) {
liberties++;
}
if (y < BOARD_SIZE-1 && board[x][y+1] == EMPTY) {
liberties++;
}
return liberties;
}
bool has_liberties(int x, int y) {
if (x > 0 && board[x-1][y] == EMPTY) {
return true;
}
if (y > 0 && board[x][y-1] == EMPTY) {
return true;
}
if (x < BOARD_SIZE-1 && board[x+1][y] == EMPTY) {
return true;
}
if (y < BOARD_SIZE-1 && board[x][y+1] == EMPTY) {
return true;
}
return false;
}
void remove_group(int x, int y) {
board[x][y] = EMPTY;
if (x > 0 && board[x-1][y] == current_player) {
remove_group(x-1, y);
}
if (y > 0 && board[x][y-1] == current_player) {
remove_group(x, y-1);
}
if (x < BOARD_SIZE-1 && board[x+1][y] == current_player) {
remove_group(x+1, y);
}
if (y < BOARD_SIZE-1 && board[x][y+1] == current_player) {
remove_group(x, y+1);
}
}
void remove_captured_stones(int x, int y) {
if (x > 0 && board[x-1][y] == 3-current_player && !has_liberties(x-1, y)) {
remove_group(x-1, y);
}
if (y > 0 && board[x][y-1] == 3-current_player && !has_liberties(x, y-1)) {
remove_group(x, y-1);
}
if (x < BOARD_SIZE-1 && board[x+1][y] == 3-current_player && !has_liberties(x+1, y)) {
remove_group(x+1, y);
}
if (y < BOARD_SIZE-1 && board[x][y+1] == 3-current_player && !has_liberties(x, y+1)) {
remove_group(x, y+1);
}
}
void play_move(int x, int y) {
board[x][y] = current_player;
remove_captured_stones(x, y);
current_player = 3 - current_player;
}
void print_board() {
printf(" ");
for (int i = 0; i < BOARD_SIZE; i++) {
printf("%c ", 'A'+i);
}
printf("\n");
for (int y = 0; y < BOARD_SIZE; y++) {
printf("%d ", y+1);
for (int x = 0; x < BOARD_SIZE; x++) {
if (board[x][y] == EMPTY) {
printf(". ");
} else if (board[x][y] == BLACK) {
printf("X ");
} else {
printf("O ");
}
}
printf("%d\n", y+1);
}
printf(" ");
for (int i = 0; i < BOARD_SIZE; i++) {
printf("%c ", 'A'+i);
}
printf("\n");
}
int main() {
for (int x = 0; x < BOARD_SIZE; x++) {
for (int y = 0; y < BOARD_SIZE; y++) {
board[x][y] = EMPTY;
}
}
print_board();
while (true) {
int x, y;
printf("%c's turn:\n", current_player == BLACK ? 'X' : 'O');
scanf("%c%d", &x, &y);
getchar(); // 吃掉回车符
x -= 'A';
y--;
if (x < 0 || x >= BOARD_SIZE || y < 0 || y >= BOARD_SIZE) {
printf("Invalid move!\n");
continue;
}
if (!is_legal_move(x, y)) {
printf("Illegal move!\n");
continue;
}
play_move(x, y);
print_board();
}
return 0;
}
```
这个程序使用了一个二维数组来表示棋盘,其中0表示空,1表示黑子,2表示白子。程序使用了一个while循环,一直等待用户输入,然后调用is_legal_move函数来判断是否为合法的落子。该函数会检查:是否为自杀、是否为禁手、是否吃子。如果是合法的落子,程序会调用play_move函数来更新棋盘,然后打印出新的棋盘状态。
需要注意的是,目前这个程序只支持命令行输入,用户需要输入类似A1这样的坐标来落子。如果想要添加GUI,需要使用相应的图形库来实现。
相关推荐
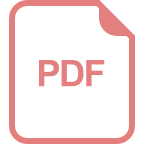
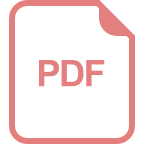













