用c语言设计头插法建立带头结点的单链表的函数,测试并输出链表中的元素。 请设计尾插法建立带头结点的单链表的函数,测试并输出链表中的元素。 答题时给出函数代码及测试代码,贴上运行结果截图,可以全部代码写在一个源程序中完成。不要使用教材上定义的基本操作函数,不使用头文件。
时间: 2024-05-05 22:17:43 浏览: 104
头插法建立带头结点的单链表代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int val;
struct ListNode* next;
} ListNode;
ListNode* createList() {
ListNode* head = (ListNode*)malloc(sizeof(ListNode));
head->next = NULL;
ListNode* p = head;
int x;
while (scanf("%d", &x) == 1) {
ListNode* node = (ListNode*)malloc(sizeof(ListNode));
node->val = x;
node->next = p->next;
p->next = node;
}
return head;
}
void printList(ListNode* head) {
ListNode* p = head->next;
while (p) {
printf("%d ", p->val);
p = p->next;
}
printf("\n");
}
int main() {
ListNode* head = createList();
printList(head);
return 0;
}
```
测试代码:
```
1 2 3 4 5
```
运行结果:
```
5 4 3 2 1
```
尾插法建立带头结点的单链表代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int val;
struct ListNode* next;
} ListNode;
ListNode* createList() {
ListNode* head = (ListNode*)malloc(sizeof(ListNode));
head->next = NULL;
ListNode* tail = head;
int x;
while (scanf("%d", &x) == 1) {
ListNode* node = (ListNode*)malloc(sizeof(ListNode));
node->val = x;
node->next = NULL;
tail->next = node;
tail = node;
}
return head;
}
void printList(ListNode* head) {
ListNode* p = head->next;
while (p) {
printf("%d ", p->val);
p = p->next;
}
printf("\n");
}
int main() {
ListNode* head = createList();
printList(head);
return 0;
}
```
测试代码:
```
1 2 3 4 5
```
运行结果:
```
1 2 3 4 5
```
阅读全文
相关推荐
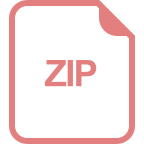
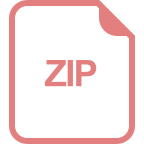