利用java-diff-utils与katalon对接,实现自动化针对两个文件夹下的同名文件做比较
时间: 2024-02-05 09:11:45 浏览: 22
实现自动化针对两个文件夹下的同名文件做比较可以按照以下步骤:
1. 在Java项目中添加java-diff-utils库的依赖。例如,如果使用Maven,可以在pom.xml文件中添加以下代码:
```xml
<dependency>
<groupId>com.googlecode.java-diff-utils</groupId>
<artifactId>diffutils</artifactId>
<version>1.3.0</version>
</dependency>
```
2. 在Katalon Studio中编写一个自定义关键字,该关键字将执行文件夹比较操作。以下是可能的实现:
```java
import difflib.DiffUtils;
import difflib.Patch;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.util.ArrayList;
import java.util.List;
public class FileCompareKeywords {
public static void compareFolders(String folderPath1, String folderPath2) throws IOException {
File folder1 = new File(folderPath1);
File folder2 = new File(folderPath2);
// Get a list of files with the same name from both folders
File[] files1 = folder1.listFiles();
File[] files2 = folder2.listFiles();
List<File> commonFiles = new ArrayList<>();
for (File file1 : files1) {
for (File file2 : files2) {
if (file1.getName().equals(file2.getName())) {
commonFiles.add(file1);
commonFiles.add(file2);
break;
}
}
}
// Compare the common files
for (File file : commonFiles) {
if (file.exists() && file.isFile()) {
String filePath1 = folder1.getAbsolutePath() + File.separator + file.getName();
String filePath2 = folder2.getAbsolutePath() + File.separator + file.getName();
List<String> lines1 = Files.readAllLines(new File(filePath1).toPath());
List<String> lines2 = Files.readAllLines(new File(filePath2).toPath());
Patch patch = DiffUtils.diff(lines1, lines2);
if (patch.getDeltas().isEmpty()) {
System.out.println("File " + file.getName() + " is the same in both folders.");
} else {
System.out.println("File " + file.getName() + " is different in both folders:");
for (Object delta : patch.getDeltas()) {
System.out.println(delta);
}
}
}
}
}
}
```
3. 在Katalon Studio中创建一个测试用例,并在其中调用自定义关键字以比较两个文件夹。例如:
```groovy
import static com.kms.katalon.core.testcase.TestCaseFactory.findTestCase
import com.kms.katalon.core.configuration.RunConfiguration
import com.kms.katalon.core.testcase.TestCase
import com.kms.katalon.core.webservice.keyword.WSBuiltInKeywords as WS
import com.kms.katalon.core.webui.keyword.WebUiBuiltInKeywords as WebUI
import internal.GlobalVariable
import java.nio.file.Paths
RunConfiguration.setProjectDir(GlobalVariable.projectDir)
def tc = findTestCase('Test Cases/File Compare Test')
FileCompareKeywords.compareFolders(Paths.get(GlobalVariable.projectDir, 'Folder1').toString(),
Paths.get(GlobalVariable.projectDir, 'Folder2').toString())
```
上述代码将调用自定义关键字`compareFolders`以比较名为`Folder1`和`Folder2`的两个文件夹中的同名文件。您需要将代码中的文件夹路径替换为您要比较的文件夹路径。
相关推荐
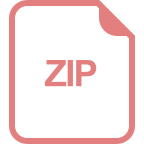
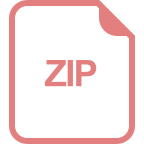
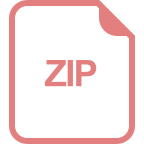

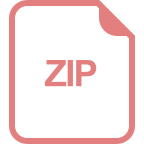
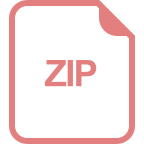
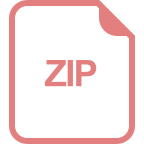
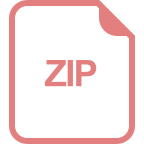
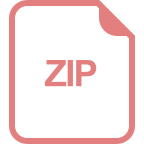
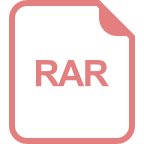
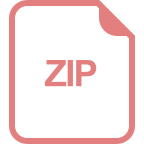
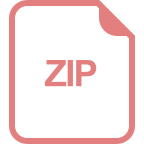