在qt中,需要保存三个表格的数据在同一个excel文件里,如何实现,当数据很大时,如何实现,需要写入的速度快一点,给IC出代码
时间: 2024-02-26 17:57:28 浏览: 85
可以使用 QAxObject 类来操作 Excel 文件,将三个表格的数据写入同一个工作簿中的不同工作表。如果数据很大,可以使用多线程来提高写入速度。
以下是示例代码:
```cpp
#include <QAxObject>
#include <QThread>
#include <QMutex>
#include <QWaitCondition>
#include <QDateTime>
#include <QDebug>
// 定义一个线程安全的队列
template<class T>
class ThreadSafeQueue {
public:
ThreadSafeQueue() : m_stop(false) {}
void push(const T& value) {
QMutexLocker lock(&m_mutex);
m_queue.enqueue(value);
m_cond.wakeOne();
}
bool pop(T& value) {
QMutexLocker lock(&m_mutex);
while (m_queue.isEmpty() && !m_stop)
m_cond.wait(&m_mutex);
if (m_stop)
return false;
value = m_queue.dequeue();
return true;
}
void stop() {
QMutexLocker lock(&m_mutex);
m_stop = true;
m_cond.wakeAll();
}
private:
QMutex m_mutex;
QWaitCondition m_cond;
bool m_stop;
QQueue<T> m_queue;
};
// 定义一个写入线程
class WriterThread : public QThread {
public:
WriterThread(QAxObject* excel, const QString& sheetName, ThreadSafeQueue<QList<QList<QVariant>>*>& queue)
: m_excel(excel), m_sheetName(sheetName), m_queue(queue) {}
void run() override {
QAxObject* workbook = m_excel->querySubObject("ActiveWorkbook");
QAxObject* worksheet = workbook->querySubObject("Worksheets(QString)", m_sheetName);
worksheet->activate();
while (true) {
QList<QList<QVariant>>* data = nullptr;
if (!m_queue.pop(data))
break;
int row = 1;
int col = 1;
for (const auto& rowValues : *data) {
for (const auto& value : rowValues) {
worksheet->querySubObject("Cells(int,int)", row, col)->setProperty("Value", value);
col++;
}
row++;
col = 1;
}
delete data;
}
workbook->dynamicCall("Save()");
workbook->dynamicCall("Close()");
}
private:
QAxObject* m_excel;
QString m_sheetName;
ThreadSafeQueue<QList<QList<QVariant>>*>& m_queue;
};
void saveToExcel(const QString& fileName, const QList<QStringList>& sheetNames, const QList<QList<QList<QVariant>>>& data) {
QAxObject excel("Excel.Application");
excel.setProperty("Visible", false);
QAxObject* workbooks = excel.querySubObject("Workbooks");
QAxObject* workbook = workbooks->querySubObject("Add()");
for (int i = 0; i < sheetNames.size(); ++i) {
QString sheetName = sheetNames[i];
QList<QList<QVariant>>* sheetData = new QList<QList<QVariant>>(data[i]);
ThreadSafeQueue<QList<QList<QVariant>>*> queue;
WriterThread* writerThread = new WriterThread(&excel, sheetName, queue);
writerThread->start();
for (int j = 0; j < sheetData->size(); ++j) {
if (j % 1000 == 0) {
// 将数据分段写入队列中
queue.push(new QList<QList<QVariant>>(sheetData->mid(j, 1000)));
}
}
queue.push(new QList<QList<QVariant>>());
writerThread->wait();
delete writerThread;
delete sheetData;
workbook->dynamicCall("Sheets(int)", i + 1)->setProperty("Name", sheetName);
}
workbook->dynamicCall("SaveAs(const QString&)", QDir::toNativeSeparators(fileName));
workbook->dynamicCall("Close()");
excel.dynamicCall("Quit()");
}
```
使用方法如下:
```cpp
QList<QStringList> sheetNames = {{"Sheet1"}, {"Sheet2"}, {"Sheet3"}};
QList<QList<QList<QVariant>>> data = {{{{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}},
{{{"a", "b", "c"}, {"d", "e", "f"}, {"g", "h", "i"}}},
{{{QDateTime::currentDateTime(), QDateTime::currentDateTime(), QDateTime::currentDateTime()},
{QDateTime::currentDateTime(), QDateTime::currentDateTime(), QDateTime::currentDateTime()},
{QDateTime::currentDateTime(), QDateTime::currentDateTime(), QDateTime::currentDateTime()}}}};
saveToExcel("test.xlsx", sheetNames, data);
```
其中,sheetNames 表示工作表的名称,data 表示要写入的数据,每个元素表示一个工作表的数据。
阅读全文
相关推荐
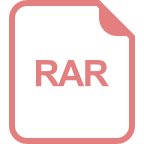
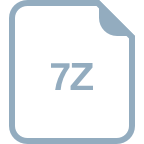
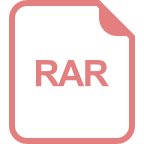
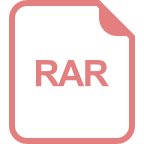
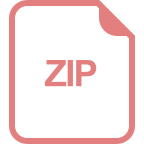
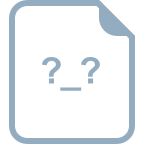
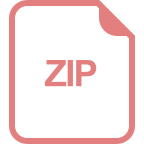
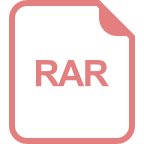
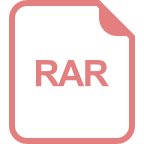
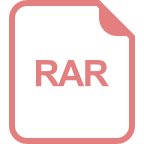
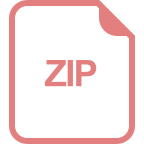
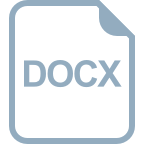
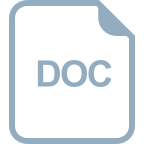
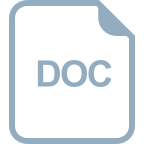
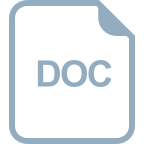
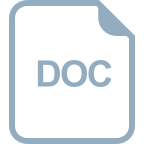
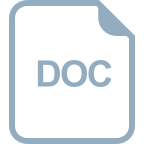