Linux 设计两个程序,分别求三边形面积和圆面积,再设计一个程序,该程序中需要创建两个子进程,两个子进程分别执行以上两个程序,子进程执行完后,父进程输出相关提示信息
时间: 2023-12-12 16:05:28 浏览: 81
以下是三个程序的代码实现,分别是求三角形面积、圆面积和父进程:
1. 求三角形面积的程序:
```c
#include <stdio.h>
int main() {
float a, b, c, s, area;
printf("Please input the length of three sides of the triangle: ");
scanf("%f%f%f", &a, &b, &c);
s = (a + b + c) / 2;
area = sqrt(s * (s-a) * (s-b) * (s-c));
printf("The area of the triangle is: %.2f\n", area);
return 0;
}
```
2. 求圆面积的程序:
```c
#include <stdio.h>
int main() {
float r, area;
printf("Please input the radius of the circle: ");
scanf("%f", &r);
area = 3.1415926 * r * r;
printf("The area of the circle is: %.2f\n", area);
return 0;
}
```
3. 父进程程序,创建两个子进程并执行以上两个程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid1, pid2;
int status1, status2;
pid1 = fork();
if (pid1 < 0) {
perror("fork error");
exit(1);
} else if (pid1 == 0) {
// 子进程1执行三角形面积程序
execl("./triangle", "./triangle", NULL);
} else {
pid2 = fork();
if (pid2 < 0) {
perror("fork error");
exit(1);
} else if (pid2 == 0) {
// 子进程2执行圆面积程序
execl("./circle", "./circle", NULL);
} else {
// 父进程等待两个子进程执行完毕
waitpid(pid1, &status1, 0);
waitpid(pid2, &status2, 0);
// 输出提示信息
printf("The two programs have been executed successfully!\n");
}
}
return 0;
}
```
以上三个程序需要分别编译成三个可执行文件 `triangle`、`circle` 和 `parent`,然后在 Linux 终端中运行 `./parent` 命令即可。
阅读全文
相关推荐
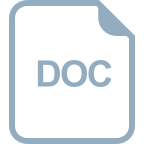
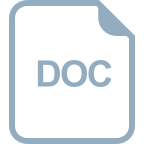
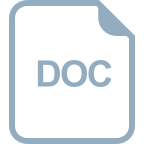




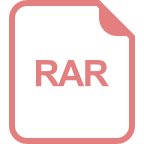
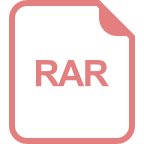
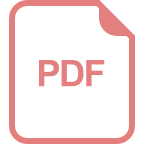
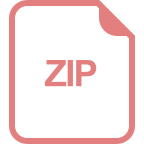
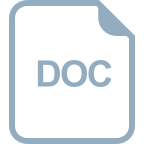




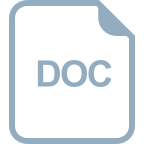
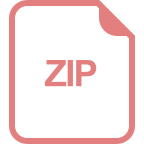