编写函数deln,具有删除链表中第n个结点的功能。再编写主函数,按输入顺序建立不带头结点的职工信息单链表,然后调用del函数删除某个职工的信息,并输出删除后的职工信息表中的职工信息。假设链表结构如下: struct staff { char num[6]; //职工工号 char name[20]; //职工姓名 double wage; //职工工资 };
时间: 2024-02-11 17:09:26 浏览: 30
以下是C++版本的代码实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
const int MAX_NUM = 6;
const int MAX_NAME = 20;
struct staff {
char num[MAX_NUM]; //职工工号
char name[MAX_NAME]; //职工姓名
double wage; //职工工资
struct staff *next; //指向下一个职工的指针
};
struct staff *create_staff(char *num, char *name, double wage) {
struct staff *s = new staff;
strcpy(s->num, num);
strcpy(s->name, name);
s->wage = wage;
s->next = NULL;
return s;
}
struct staff *create_list() {
struct staff *head = NULL, *tail = NULL;
char num[MAX_NUM], name[MAX_NAME];
double wage;
int n, i;
cout << "请输入职工数量:";
cin >> n;
cout << "请分别输入职工工号、姓名和工资:" << endl;
for (i = 0; i < n; i++) {
cin >> num >> name >> wage;
struct staff *s = create_staff(num, name, wage);
if (tail == NULL) {
head = s;
tail = s;
} else {
tail->next = s;
tail = s;
}
}
return head;
}
struct staff *deln(struct staff *head, int n) {
if (head == NULL) {
cout << "链表为空!" << endl;
return NULL;
}
if (n <= 0) {
cout << "无效的职工编号!" << endl;
return head;
}
if (n == 1) {
struct staff *p = head->next;
delete head;
return p;
}
struct staff *p = head;
int i;
for (i = 1; i < n - 1 && p != NULL; i++) {
p = p->next;
}
if (p == NULL || p->next == NULL) {
cout << "无效的职工编号!" << endl;
return head;
}
struct staff *q = p->next;
p->next = q->next;
delete q;
return head;
}
void print_list(struct staff *head) {
cout << "职工信息表:" << endl;
cout << " 编号 姓名 工资" << endl;
struct staff *p = head;
while (p != NULL) {
cout << p->num << " " << p->name << " " << p->wage << endl;
p = p->next;
}
}
int main() {
struct staff *head = create_list();
print_list(head);
int n;
cout << "请输入要删除的职工编号:";
cin >> n;
head = deln(head, n);
print_list(head);
return 0;
}
```
这个实现与前面的C语言实现类似,只是使用了C++的动态内存分配和输入输出方式。在 `create_staff` 函数中,我们使用了 `new` 关键字来动态分配内存,以创建一个新的职工信息对象。在 `deln` 函数中,我们使用了 `delete` 关键字来释放动态分配的内存。另外,我们使用了 `cout` 和 `cin` 来进行输入输出,这比C语言中的 `printf` 和 `scanf` 更加方便和安全。
需要注意的是,在C++中,我们可以使用 `std::string` 代替字符数组,来更加方便地处理字符串。此外,我们还可以使用STL提供的链表容器(`std::list`)来实现链表操作,这样可以避免手动管理内存,提高程序的安全性和可读性。
相关推荐
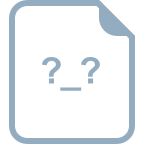
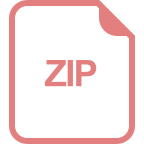
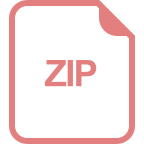

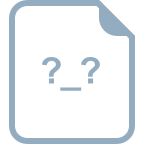
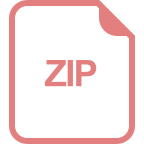
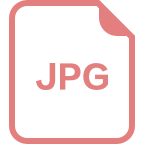
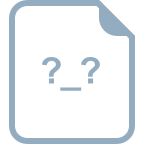
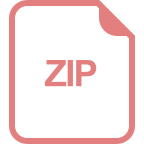