查询功能:分为学生基本情况查询和成绩查询两种 A:学生基本情况查询:输入一个学号,查出此生的基本信息并显示输出。 B:成绩查询:输入一个学号时,查询出此生的所有课程情况,格式如下: 学 号:xx 姓 名:xxxxx 课程编号:xxx 课程名称:xxxxx 综合成绩:xxxx 实得学分: xx 课程编号:xxx 课程名称:xxxxx 综合成绩:xxxx 实得学分: xx 课程编号:xxx 课程名称:xxxxx 综合成绩:xxxx 实得学分: xx 共修:xx科,实得总学分为: xxx
时间: 2024-02-15 16:04:11 浏览: 207
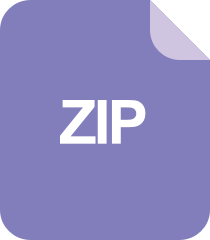
guanlixitong.zip_学生成绩_成绩查询系统
为了实现查询功能,需要先读取保存学生信息和课程成绩的文件,然后根据输入的学号查找对应的学生信息和课程成绩信息,最后输出查询结果。
以下是一个简单的示例代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生信息结构体
typedef struct {
char id[50];
char name[50];
int age;
} Student;
// 定义课程信息结构体
typedef struct {
char id[50];
char name[50];
int score;
int credit;
int is_common;
} Course;
// 从文件中读取学生信息
void read_students(const char* filename, Student students[], int* count) {
FILE* fp = fopen(filename, "r");
if (fp == NULL) {
printf("Failed to open file %s\n", filename);
exit(1);
}
*count = 0;
while (!feof(fp)) {
fscanf(fp, "%s %s %d", students[*count].id, students[*count].name, &students[*count].age);
(*count)++;
}
fclose(fp);
}
// 从文件中读取课程信息
void read_courses(const char* filename, Course courses[], int* count) {
FILE* fp = fopen(filename, "r");
if (fp == NULL) {
printf("Failed to open file %s\n", filename);
exit(1);
}
*count = 0;
while (!feof(fp)) {
fscanf(fp, "%s %s %d %d %d", courses[*count].id, courses[*count].name, &courses[*count].score, &courses[*count].credit, &courses[*count].is_common);
(*count)++;
}
fclose(fp);
}
// 根据学号查找学生信息
void find_student_by_id(const char* filename, const char* student_id, Student* student) {
Student students[100];
int count;
read_students(filename, students, &count);
for (int i = 0; i < count; i++) {
if (strcmp(students[i].id, student_id) == 0) {
*student = students[i];
return;
}
}
printf("Cannot find student with id %s\n", student_id);
exit(1);
}
// 根据学号查找课程成绩信息
void find_scores_by_id(const char* filename, const char* student_id, Course courses[], int* count, int* common_count, int* common_credit, int* total_credit) {
FILE* fp = fopen(filename, "r");
if (fp == NULL) {
printf("Failed to open file %s\n", filename);
exit(1);
}
*count = 0;
*common_count = 0;
*common_credit = 0;
*total_credit = 0;
char id[50];
while (!feof(fp)) {
fscanf(fp, "%s", id);
if (strcmp(id, student_id) == 0) {
char course_id[50];
int score, credit, is_common;
while (fscanf(fp, "%s %d %d %d", course_id, &score, &credit, &is_common) == 4) {
strcpy(courses[*count].id, course_id);
courses[*count].score = score;
courses[*count].credit = credit;
courses[*count].is_common = is_common;
(*count)++;
if (is_common) {
(*common_count)++;
(*common_credit) += credit;
}
(*total_credit) += credit;
}
break;
} else {
// 跳过该学生的成绩信息
char line[100];
fgets(line, 100, fp);
}
}
fclose(fp);
}
int main() {
// 输入学号
char student_id[50];
printf("Please input student id: ");
scanf("%s", student_id);
// 查找学生信息
Student student;
find_student_by_id("students.txt", student_id, &student);
// 输出学生信息
printf("学 号:%s\n", student.id);
printf("姓 名:%s\n", student.name);
// 查找课程成绩信息
Course courses[100];
int count, common_count, common_credit, total_credit;
find_scores_by_id("scores.txt", student_id, courses, &count, &common_count, &common_credit, &total_credit);
// 输出课程成绩信息
printf("共修:%d科,实得总学分为:%d\n", common_count, common_credit);
for (int i = 0; i < count; i++) {
printf("\n课程编号:%s\n", courses[i].id);
printf("课程名称:%s\n", courses[i].name);
printf("综合成绩:%d\n", courses[i].score);
printf("实得学分:%d\n", courses[i].credit);
}
return 0;
}
```
请注意,在这个示例代码中,我们假设学生信息保存在students.txt文件中,每一行为一个学生信息,以空格分隔;课程成绩信息保存在scores.txt文件中,每一行为一个学生的所有课程成绩信息,以空格分隔。如果您的文件格式不同,需要适当修改代码以适应不同的文件格式。
阅读全文
相关推荐
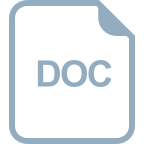
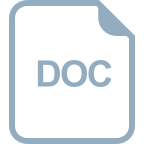
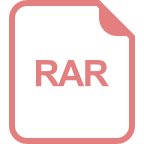
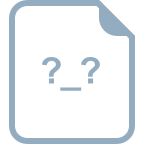
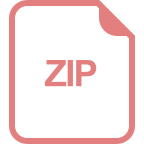
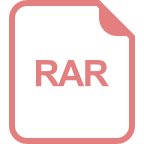
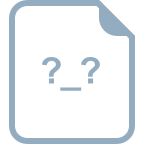
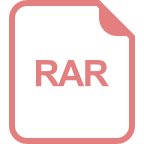
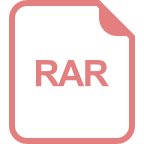
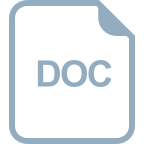
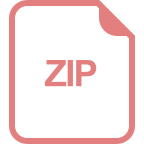
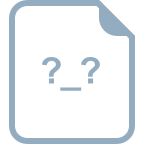
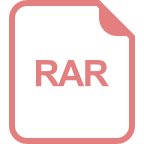
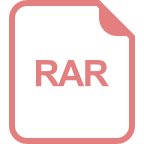
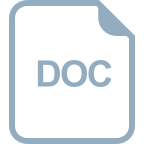
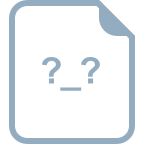
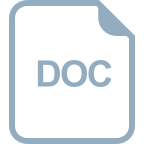
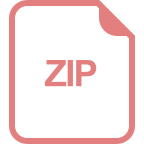