使用python中的tinker进行GUI设计的详细流程概述
时间: 2024-02-16 15:04:23 浏览: 278
首先,需要导入tkinter模块:
```python
import tkinter as tk
```
然后,创建一个主窗口:
```python
root = tk.Tk()
```
接着,可以设置窗口的标题、大小、位置等属性:
```python
root.title("My Application")
root.geometry("500x500") # 设置窗口大小
root.resizable(False, False) # 禁止窗口大小调整
root.configure(bg="#F0F0F0") # 设置背景颜色
```
在主窗口中,可以添加各种控件,例如标签、按钮、文本框等:
```python
label = tk.Label(root, text="Hello, World!")
button = tk.Button(root, text="Click Me!")
entry = tk.Entry(root)
```
然后,可以使用pack()或grid()方法将控件添加到窗口中:
```python
label.pack()
button.pack()
entry.grid(row=0, column=1)
```
最后,调用主窗口的mainloop()方法,进入事件循环,等待用户操作:
```python
root.mainloop()
```
在事件循环中,可以设置各种事件处理函数,例如按钮点击事件、键盘事件等。这些事件处理函数可以使用bind()方法或command参数进行绑定:
```python
def handle_click():
print("Button Clicked!")
button = tk.Button(root, text="Click Me!", command=handle_click)
entry.bind("<Return>", handle_keypress)
```
以上就是使用tkinter进行GUI设计的基本流程。需要注意的是,GUI设计需要考虑用户交互的便捷性、界面的美观性和易用性等方面,因此需要仔细设计和调试。
阅读全文
相关推荐





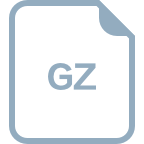
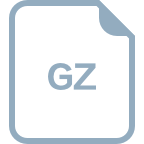
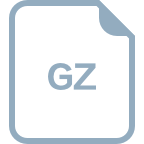









